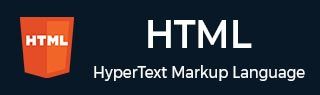
- HTML 教程
- HTML - 首页
- HTML - 路线图
- HTML - 简介
- HTML - 历史与演变
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块
- HTML - 样式表
- HTML - 格式化
- HTML - 引述
- HTML - 注释
- HTML - 颜色
- HTML - 图像
- HTML - 图像地图
- HTML - 内联框架
- HTML - 短语元素
- HTML - 元标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表格标题和说明
- HTML - 表格样式
- HTML - 表格 Colgroup
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图像链接
- HTML - 邮件链接
- HTML 颜色名称和值
- HTML - 颜色名称
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 页眉
- HTML - 头元素
- HTML - 添加 Favicon
- HTML - Javascript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - 使用 CSS 进行布局
- HTML - 响应式设计
- HTML - 符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - Canvas
- HTML API
- HTML - Geolocation API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 其他
- HTML - 文档对象模型 (DOM)
- HTML - MathML
- HTML - 微数据
- HTML - IndexedDB
- HTML - Web 消息传递
- HTML - Web CORS
- HTML - Web RTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - 网页幻灯片
- HTML 工具
- HTML - Velocity Draw
- HTML - 二维码
- HTML - Modernizer
- HTML - 验证
- HTML - 颜色拾取器
- HTML 参考
- HTML - 速查表
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 码
- ASCII 码表查找
- HTML - 颜色名称
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- 语言 ISO 代码
- HTML - 字符编码
- HTML - 已弃用标签
- HTML 资源
- HTML - 快速指南
- HTML - 有用资源
- HTML - 颜色代码生成器
- HTML - 在线编辑器
HTML - DOM 元素 scrollTop 属性
**scrollTop** 属性允许您访问和更新元素内容垂直滚动的距离,以像素为单位测量元素顶边缘的距离。
语法
设置 scrollLeft 属性值element.scrollTop = pixelValue;获取 scrollLeft 属性值
element.scrollTop;
属性值
值 | 描述 |
---|---|
pixelValue | 像素值,设置元素的垂直滚动位置。
|
返回值
scrollTop 属性返回一个整数,表示以像素为单位的垂直滚动位置。
HTML DOM 元素 'scrollTop' 属性示例
以下是一些示例,展示了如何使用 'scrollTop' 属性调整 HTML DOM 中元素的垂直滚动位置。
显示实时滚动位置
此示例包含一个具有固定高度和可滚动内容的 **<div>** 元素。在事件监听器中使用 scrollTop 属性,它会在您滚动时动态更新 元素内的滚动位置。
<!DOCTYPE html> <html lang="en"> <head> <style> #myDIV { height: 100px; width: 200px; overflow: auto; border: 1px solid black; padding: 10px; } </style> </head> <body> <h1>HTML - DOM Element</h1> <div id="myDIV"> <h2>scrollTop Property</h2> <p>Scroll Me!!<p> <p>To get the scrolled position.</p> <p> Some content inside the scrollable container. </p> </div> <p> Scroll position:<span id="scrollPos"></span> </p> <script> // Get the element const m= document.getElementById('myDIV'); // Update the scroll position on scroll m.addEventListener('scroll', function() { const sp = m.scrollTop; document.getElementById('scrollPos').textContent = sp + 'px'; }); </script> </body> </html>
单击按钮垂直滚动容器
此示例包含一个按钮,单击该按钮会将 **<body>** 元素的整个内容滚动到特定位置。它通过每次单击按钮时分别在水平和垂直方向上添加 30 像素和 10 像素来调整滚动位置。
<!DOCTYPE html> <html lang="en"> <head> <style> .con { height: 300px; width: 80%; overflow: auto; border: 1px solid black; } .content { height: 800px; } </style> </head> <body> <h1>HTML - DOM Element</h1> <h2>scrollTop Property</h2> <div class="con"> <div class="content"> <p> Scroll Me by Clicking the Button!!. </p> <p>Below!.</p> <p>This is some content.</p> </div> </div> <br> <br> <button onclick="scrollContainer()"> Scroll Container </button> <script> function scrollContainer() { var con = document.querySelector('.con'); con.scrollLeft += 30; con.scrollTop += 10; } </script> </body> </html>
跟踪和显示垂直滚动位置
此示例演示了如何使用 scrollTop 属性实时跟踪和显示可滚动 <div> 元素的垂直滚动位置。
<!DOCTYPE html> <html lang="en"> <head> <style> #s-div { height: 200px; overflow-y: scroll; border: 1px solid #ccc; } .content { height: 1000px; } #scroll-info { margin-top: 10px; } </style> </head> <body> <h1>HTML - DOM Element</h1> <h2>scrollTop Property</h2> <div id="s-div"> <div class="content"> Scroll me to check whether we reached the bottom or not.!! </div> </div> <div id="scroll-info"> Scroll Top: <span id="st">0</span><br> Reached Bottom: <span id="br">No</span> </div> <script> var sd = document.getElementById('s-div'); var ste = document.getElementById('st'); var bre = document.getElementById('br'); sd.addEventListener('scroll', function() { // Get the current scrollTop value var scrollTopVal = sd.scrollTop; // Update the scroll top value display ste.textContent = scrollTopVal; // Check if user reached bottom of scroll if (scrollTopVal + sd.clientHeight >= sd.scrollHeight) { bre.textContent = 'Yes'; } else { bre.textContent = 'No'; } }); </script> </body> </html>
设置滚动位置
此示例演示了通过设置可滚动元素的可滚动位置来使用 scrollTop 属性。
<!DOCTYPE html> <html lang="en"> <head> <style> .scr { height: 120px; width: 200px; overflow-y: scroll; border: 1px solid #ccc; } </style> </head> <body> <h1>HTML - DOM Element</h1> <div class="scr" id="scrDiv"> <h2>scrollTop Property</h2> <p>This example allows <br>you to set the <br> scroll position to 50 pixel, <br> which upon clicking <br>the button will adjust the scrollable position to <br>50 pixel. </p> </div> <script> // Set the scroll position function setScrollPosition() { var ele=document.getElementById('scrDiv'); // Set the scroll position to 50 pixels ele.scrollTop = 50; } </script> <br> <button onclick="setScrollPosition()"> Set Scroll Position to 50px </button> </body> </html>
滚动时背景颜色更改
此示例使用 scrollTop 属性。运行此代码后尝试滚动。您将看到滚动超过页面顶部 100 像素后背景颜色变为红色。
<!DOCTYPE html> <html lang="en"> <head> <style> body { margin: 0; height: 2000px; } header { background-color: #333; color: #fff; text-align: center; padding: 10px 0; } .content { margin-top: 100px; padding: 20px; text-align: center; font-size: 18px; } .highlight { background-color: red; } </style> </head> <body> <header> <h1>ScrollTop Event Example</h1> </header> <div class="content" id="myContent"> <p> Scroll down to see the effect. Once you scroll past 100 pixels from the top, this paragraph will have a yellow background. </p> </div> <script> window.onscroll = function() { myFunction() }; function myFunction() { var scrollPosition = document.documentElement.scrollTop || document.body.scrollTop; if (scrollPosition > 100) { document.getElementById ("myContent").classList.add("highlight"); } else { document.getElementById ("myContent").classList.remove ("highlight"); } } </script> </body> </html>
使用 'scrollTop' 属性创建滚动到顶部指示器
此示例演示了如何使用 scrollTop 属性创建当用户向下滚动页面时出现的滚动到顶部指示器。单击它会将页面平滑地滚动回顶部。
<!DOCTYPE html> <html lang="en"> <head> <style> body { height: 2000px; } .scr-ind { position: fixed; bottom: 20px; right: 20px; background-color: rgba(0, 0, 0, 0.5); color: white; padding: 10px 20px; border-radius: 5px; display: none; } </style> </head> <body> <h1>HTML - DOM Element</h1> <h2>scrollTop Property </h2> <p>Scroll down to see top indicator!</p> <div class="scr-ind" id="scrollI">Top</div> <script> // Display scroll indicator when scrolling down window.onscroll = function() { var scr=document.getElementById("scrollI"); if (document.body.scrollTop > 200 || document.documentElement.scrollTop > 200){ scr.style.display = "block"; } else { scr.style.display = "none"; } }; // Scroll to top when indicator is clicked document.getElementById("scrollI").addEventListener ("click", function() { // For Chrome, Firefox, IE and Opera document.documentElement.scrollTop = 0; }); </script> </body> </html>
支持的浏览器
属性 | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
scrollTop | 是 | 是 | 是 | 是 | 是 |
html_dom_element_reference.htm
广告