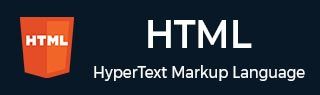
- HTML 教程
- HTML - 首页
- HTML - 路线图
- HTML - 简介
- HTML - 历史与演变
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块
- HTML - 样式表
- HTML - 格式化
- HTML - 引用
- HTML - 注释
- HTML - 颜色
- HTML - 图片
- HTML - 图片地图
- HTML - 内联框架
- HTML - 短语元素
- HTML - 元标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表头和标题
- HTML - 表格样式
- HTML - 表格 Colgroup
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 邮件链接
- HTML 颜色名称和值
- HTML - 颜色名称
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 头部
- HTML - Head 元素
- HTML - 添加 Favicon
- HTML - Javascript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - 使用 CSS 进行布局
- HTML - 响应式
- HTML - 符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - Canvas
- HTML API
- HTML - 地理位置 API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 杂项
- HTML - 文档对象模型 (DOM)
- HTML - MathML
- HTML - 微数据
- HTML - IndexedDB
- HTML - Web 消息传递
- HTML - Web CORS
- HTML - Web RTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - 网页幻灯片
- HTML 工具
- HTML - Velocity Draw
- HTML - 二维码
- HTML - Modernizer
- HTML - 验证
- HTML - 颜色拾取器
- HTML 参考
- HTML - 速查表
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 码
- ASCII 表格查找
- HTML - 颜色名称
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- 语言 ISO 代码
- HTML - 字符编码
- HTML - 已弃用的标签
- HTML 资源
- HTML - 快速指南
- HTML - 有用资源
- HTML - 颜色代码生成器
- HTML - 在线编辑器
HTML - DOM 元素 toString() 方法
**toString()** 方法允许我们将 HTML 元素转换为字符串格式,而不会更改原始元素。它通常显示其类型为 [object Object]。
语法
object.toString();
参数
此方法不接受任何参数。
返回值
toString() 方法不会直接返回值。相反,它会自动用于将对象转换为字符串格式。
HTML DOM 元素 'toString()' 方法示例
以下是 toString() 方法的一些示例,这些示例将对象转换为字符串格式,以便在 HTML DOM 中显示或执行不同的操作。
在 HTML 元素上使用 toString() 方法
此示例显示了 toString 方法的使用。代码通过调用 toString() 函数访问 **<div>** 元素的内容,该函数将元素转换为其字符串格式并将内容更新为显示 toString() 的结果。
<!DOCTYPE html> <html lang="en"> <head> <title>toString() Method Example</title> </head> <body> <h1>HTML - DOM Element</h1> <h2>toString() Method</h2> <p>Click the button to get the result!</p> <div id="myDiv"> <p>Example content.</p> </div> <button onclick="displayToString()"> Display toString() Result </button> <div id="ot"></div> <script> function displayToString() { const m=document.getElementById('myDiv'); const opd=document.getElementById('ot'); // Using toString() on the HTML element const elementString = m.toString(); // Displaying the result opd.textContent = `toString() Result: ${elementString}`; } </script> </body> </html>
将数组转换为字符串
此示例包含一个包含元素的数组。toString() 方法用于将数组转换为字符串。然后,代码更新 **<div>** 元素的内容以显示数组作为字符串。
<!DOCTYPE html> <html lang="en"> <head> <title>toString() Example: Array</title> </head> <body> <h1>HTML - DOM Element</h1> <h2>toString() Method</h2> <p>Converting an Array to String</p> <button onclick="convertArrayToString()"> Convert Array to String </button> <div id="output"></div> <script> function convertArrayToString() { const my=['apple', 'banana', 'cherry']; const arrayString = my.toString(); const ot=document.getElementById('output'); ot.textContent = `Array as String: ${arrayString}`; } </script> </body> </html>
将数字转换为字符串
此示例显示了使用 toString() 方法将数字转换为字符串。然后,代码调用 toString() 方法并更新 **<div>** 元素的内容以显示数字作为字符串。
<!DOCTYPE html> <html lang="en"> <head> <title>Converting Number</title> </head> <body> <h1>HTML - DOM Element</h1> <h2>toString() Method</h2> <p>Converting Number to String</p> <button onclick="convertNumberToString()"> Convert Number to String </button> <div id="output"></div> <script> function convertNumberToString() { const myNumber = 12345; const numstr = myNumber.toString(); const ot=document.getElementById('output'); ot.textContent = `Number as String: ${numstr}`; } </script> </body> </html>
将对象转换为字符串
此示例显示了使用 toString() 方法将具有属性“name”和“age”的对象转换为字符串。然后,代码调用 toString() 方法并更新 **<div>** 元素的内容以显示对象作为字符串。
<!DOCTYPE html> <html lang="en"> <head> <title>Converting Object</title> </head> <body> <h1>HTML - DOM Element</h1> <h2>toString() Method</h2> <p>Converting Object to String</p> <button onclick="convertObjectToString()"> Convert Object to String </button> <div id="output"></div> <script> function convertObjectToString() { const myObject={name:'John',age:30}; const objectString=myObject.toString(); const outputDiv = document.getElementById('output'); outputDiv.textContent = `Object as String: ${objectString}`; } </script> </body> </html>
支持的浏览器
方法 | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
toString() | 是 | 是 | 是 | 是 | 是 |
html_dom_element_reference.htm
广告