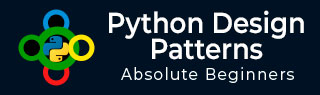
- Python 设计模式教程
- Python 设计模式 - 首页
- 简介
- Python 设计模式 - 要点
- 模型视图控制器模式
- Python 设计模式 - 单例
- Python 设计模式 - 工厂
- Python 设计模式 - 构建器
- Python 设计模式 - 原型
- Python 设计模式 - 外观
- Python 设计模式 - 命令
- Python 设计模式 - 适配器
- Python 设计模式 - 装饰器
- Python 设计模式 - 代理
- 责任链模式
- Python 设计模式 - 观察者
- Python 设计模式 - 状态
- Python 设计模式 - 策略
- Python 设计模式 - 模板
- Python 设计模式 - 轻量级
- 抽象工厂
- 面向对象
- 面向对象概念实现
- Python 设计模式 - 迭代器
- 字典
- 列表数据结构
- Python 设计模式 - 集合
- Python 设计模式 - 队列
- 字符串和序列化
- Python 中的并发
- Python 设计模式 - 反模式
- 异常处理
- Python 设计模式资源
- 快速指南
- Python 设计模式 - 资源
- 讨论
Python 设计模式 - 字典
字典是数据结构,包括键值组合。它们广泛用于替代 JSON(JavaScript 对象表示法)。字典用于 API(应用程序编程接口)编程。字典将一组对象映射到另一组对象。字典是可变的,这意味着可以根据需要随时更改它们。
如何在 Python 中实现字典?
以下程序展示了在 Python 中实现字典的基本过程,从创建到实现。
# Create a new dictionary d = dict() # or d = {} # Add a key - value pairs to dictionary d['xyz'] = 123 d['abc'] = 345 # print the whole dictionary print(d) # print only the keys print(d.keys()) # print only values print(d.values()) # iterate over dictionary for i in d : print("%s %d" %(i, d[i])) # another method of iteration for index, value in enumerate(d): print (index, value , d[value]) # check if key exist 23. Python Data Structure –print('xyz' in d) # delete the key-value pair del d['xyz'] # check again print("xyz" in d)
输出
上述程序生成以下输出 −
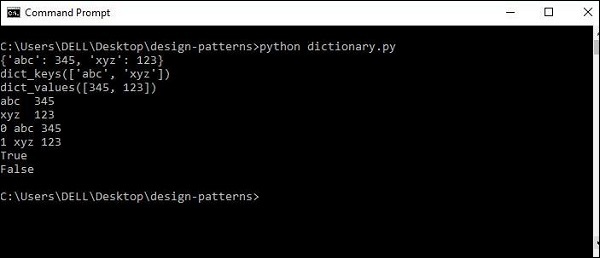
注意 −在 Python 中实现字典存在缺点。
缺点
字典不支持诸如字符串、元组和列表一类的序列数据类型的序列操作。这些属于内置映射类型。
广告