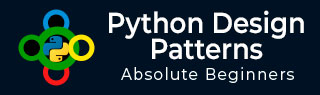
- Python 设计模式教程
- Python 设计模式-主页
- 简介
- Python 设计模式-要点
- 模型视图控制器模式
- Python 设计模式-单例模式
- Python 设计模式-工厂模式
- Python 设计模式-建造器模式
- Python 设计模式-原型模式
- Python 设计模式-外观模式
- Python 设计模式-命令模式
- Python 设计模式-适配器模式
- Python 设计模式-装饰器模式
- Python 设计模式-代理模式
- 责任链模式
- Python 设计模式-观察者模式
- Python 设计模式-状态模式
- Python 设计模式-策略模式
- Python 设计模式-模板方法模式
- Python 设计模式-享元模式
- 抽象工厂模式
- 面向对象
- 面向对象的实现概念
- Python 设计模式-迭代器模式
- 字典
- 列表数据结构
- Python 设计模式-集合模式
- Python 设计模式-队列模式
- 字符串和序列化
- Python 中的并发
- Python 设计模式-反向设计模式
- 异常处理
- Python 设计模式资源
- 快速指南
- Python 设计模式-资源
- 讨论
Python 设计模式-面向对象
面向对象的模式是最常用的模式。几乎在所有编程语言中都可以找到此模式。
如何实现面向对象的模式?
现在让我们看看如何实现面向对象的模式。
class Parrot: # class attribute species = "bird" # instance attribute def __init__(self, name, age): self.name = name self.age = age # instantiate the Parrot class blu = Parrot("Blu", 10) woo = Parrot("Woo", 15) # access the class attributes print("Blu is a {}".format(blu.__class__.species)) print("Woo is also a {}".format(woo.__class__.species)) # access the instance attributes print("{} is {} years old".format( blu.name, blu.age)) print("{} is {} years old".format( woo.name, woo.age))
输出
以上程序生成以下输出
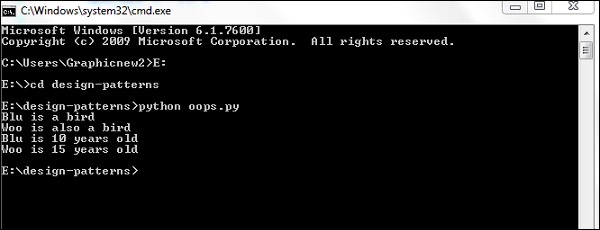
说明
该代码包括类属性和实例属性,它们会根据输出的要求进行打印。面向对象的模式由各种特性组成。下一章将对这些特性进行说明。
广告