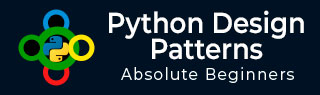
- Python 设计模式教程
- Python 设计模式 - 首页
- 介绍
- Python 设计模式 - 要点
- 模型-视图-控制器模式
- Python 设计模式 - 单例模式
- Python 设计模式 - 工厂模式
- Python 设计模式 - 建造者模式
- Python 设计模式 - 原型模式
- Python 设计模式 - 外观模式
- Python 设计模式 - 命令模式
- Python 设计模式 - 适配器模式
- Python 设计模式 - 装饰器模式
- Python 设计模式 - 代理模式
- 责任链模式
- Python 设计模式 - 观察者模式
- Python 设计模式 - 状态模式
- Python 设计模式 - 策略模式
- Python 设计模式 - 模板模式
- Python 设计模式 - 享元模式
- 抽象工厂模式
- 面向对象
- 面向对象概念实现
- Python 设计模式 - 迭代器模式
- 字典
- 列表数据结构
- Python 设计模式 - 集合
- Python 设计模式 - 队列
- 字符串与序列化
- Python中的并发
- Python 设计模式 - 反模式
- 异常处理
- Python 设计模式资源
- 快速指南
- Python 设计模式 - 资源
- 讨论
Python 设计模式 - 队列
队列是一种对象集合,它定义了一种简单的遵循FIFO(先进先出)和LIFO(后进先出)原则的数据结构。插入和删除操作分别称为入队和出队操作。
队列不允许随机访问其包含的对象。
如何实现FIFO原则?
以下程序有助于实现FIFO:
import Queue q = Queue.Queue() #put items at the end of the queue for x in range(4): q.put("item-" + str(x)) #remove items from the head of the queue while not q.empty(): print q.get()
输出
上述程序生成以下输出:
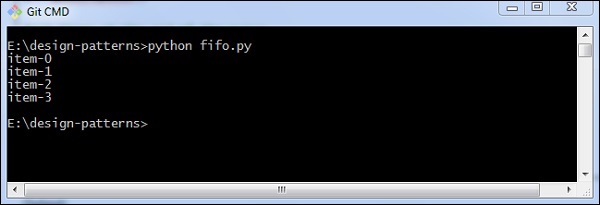
如何实现LIFO原则?
以下程序有助于实现LIFO原则:
import Queue q = Queue.LifoQueue() #add items at the head of the queue for x in range(4): q.put("item-" + str(x)) #remove items from the head of the queue while not q.empty(): print q.get()
输出
上述程序生成以下输出:
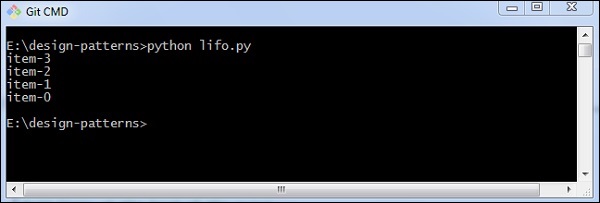
什么是优先队列?
优先队列是一种容器数据结构,它管理一组具有有序键的记录,以便快速访问具有指定数据结构中最小或最大键的记录。
如何实现优先队列?
优先队列的实现如下:
import Queue class Task(object): def __init__(self, priority, name): self.priority = priority self.name = name def __cmp__(self, other): return cmp(self.priority, other.priority) q = Queue.PriorityQueue() q.put( Task(100, 'a not agent task') ) q.put( Task(5, 'a highly agent task') ) q.put( Task(10, 'an important task') ) while not q.empty(): cur_task = q.get() print 'process task:', cur_task.name
输出
上述程序生成以下输出:
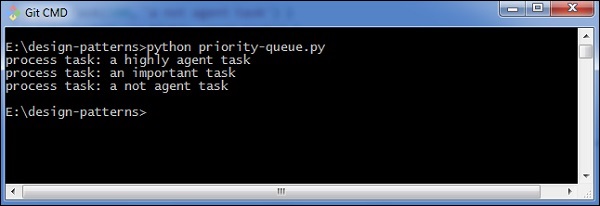
广告