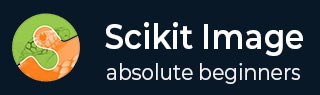
- Scikit Image 教程
- Scikit Image - 简介
- Scikit Image - 图像处理
- Scikit Image - NumPy图像
- Scikit Image - 图像数据类型
- Scikit Image - 使用插件
- Scikit Image - 图像处理
- Scikit Image - 读取图像
- Scikit Image - 写入图像
- Scikit Image - 显示图像
- Scikit Image - 图像集合
- Scikit Image - 图像堆栈
- Scikit Image - 多图像
Scikit Image - 图像数据类型
在计算机编程的上下文中,数据类型是指根据数据的属性及其可以执行的操作对数据进行分类或归类。它决定了计算机如何解释、存储和处理数据。
不同的编程语言有自己的一套数据类型,每种数据类型都有其属性。常见的数据类型包括整数、浮点数、字符、字符串、布尔值和数组。
Python Scikit Image 中的数据类型
在 scikit-image 中,图像表示为 NumPy 数组,并支持多种数据类型,也称为“dtypes”。库为 dtype 设置了某些范围,以避免图像强度失真。以下是 scikit-image 中常用的 dtypes 及其相应的范围:
- uint8 − 无符号 8 位整数,范围从 0 到 255。
- uint16 − 无符号 16 位整数,范围从 0 到 65535。
- uint32 − 无符号 32 位整数,范围从 0 到 2^32 - 1。
- float − 浮点数,通常范围从 -1 到 1 或 0 到 1。
- int8 − 有符号 8 位整数,范围从 -128 到 127。
- int16 − 有符号 16 位整数,范围从 -32768 到 32767。
- int32 − 有符号 32 位整数,范围从 -2^31 到 2^31 - 1。
请注意,即使浮点型 dtype 本身可以超过此范围,浮点图像通常应限制在 -1 到 1 的范围内。另一方面,整数 dtype 可以跨越其各自数据类型的整个范围。务必坚持这些范围,以避免数据丢失或像素强度解释错误。
图像数据类型转换函数
在 scikit-image 中,skimage.util 模块中有一些可用的函数可以转换图像数据类型并确保图像强度的正确重新缩放。这些函数旨在处理转换和重新缩放,同时保留图像的数据范围。以下是 scikit-image 中的图像数据类型转换函数:
- Img_as_float
- Img_as_ubyte
- Img_as_uint
- Img_as_int
这些函数提供了一种方便的方法来将图像转换为所需的数据类型,同时保持正确的强度范围。此外,重要的是避免直接在图像上使用 astype 函数,因为它可能会违反关于 dtype 范围的假设。相反,您可以使用上述转换函数来确保正确的 dtype 转换和强度重新缩放。
示例 1
以下示例演示了在 scikit-image 中使用astype()方法和img_as_float()函数转换图像数组的数据类型之间的区别。
from skimage import util import numpy as np # Create an image with 8-bit unsigned integers image = np.random.randint(0, 256, size=(1, 4), dtype=np.uint8) print("Image array:", image) # Convert the image to float using astype() print('Converted to float using astype :',image.astype(float)) # These float values are out of range. # Convert the image to float using img_as_float() print("Converted to float using img_as_float:",util.img_as_float(image))
输出
Image array: [[173 104 167 25]] Converted to float using astype : [[173. 104. 167. 25.]] Converted to float using img_as_float: [[0.67843137 0.40784314 0.65490196 0.09803922]]
通过使用img_as_float()函数,图像数组被正确转换为浮点数据类型,强度值在有效范围内正确缩放。
这确保了正确的数据类型转换和强度重新缩放。
示例 2
以下示例演示了如何使用skimage.util模块中的img_as_ubyte()函数将浮点图像数组转换为 8 位无符号整数表示。
from skimage import util import numpy as np # Create an image with floating point numbers image = np.array([0, 0.1, 0, 0.8, 0.3, 1], dtype=float) print("Image array:", image) # Convert the image data to 8-bit Unsigned integers print("Converted to 8-bit uint using img_as_ubyte:",util.img_as_ubyte(image))
输出
Image array: [0. 0.1 0. 0.8 0.3 1.] Converted to 8-bit uint using img_as_ubyte: [0 26 0 204 76 255]