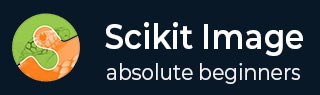
- Scikit-image 教程
- Scikit-image - 简介
- Scikit-image - 图像处理
- Scikit-image - NumPy 图像
- Scikit-image - 图像数据类型
- Scikit-image - 使用插件
- Scikit-image - 图像处理
- Scikit-image - 读取图像
- Scikit-image - 写入图像
- Scikit-image - 显示图像
- Scikit-image - 图像集合
- Scikit-image - 图像堆栈
- Scikit-image - 多张图像
Scikit-image - 写入图像
写入或保存图像在图像处理和计算机视觉任务中起着至关重要的作用。在执行图像处理操作(如裁剪、调整大小、旋转或应用各种滤镜)时,需要保存图像。
写入图像指的是将图像保存或存储为磁盘或内存中外部文件的过程。在此过程中,我们通常指定所需的格式(例如 JPEG、PNG、TIFF 等)并提供文件名或路径。
在 scikit-image 库中,io 模块提供了一个名为 imsave() 的函数,专门用于将图像写入和存储为外部文件。此函数可用于保存图像操作的结果。
io.imsave() 方法
通过使用imsave()方法,可以将图像写入具有所需文件格式、文件名和所需位置的外部文件。以下是此方法的语法:
skimage.io.imsave(fname, arr, plugin=None, check_contrast=True, **plugin_args)
该函数采用以下参数:
- fname - 表示将保存图像的文件名或路径的字符串。文件的格式从文件名的扩展名获取。
- arr - 形状为 (M,N) 或 (M,N,3) 或 (M,N,4) 的 NumPy 数组,包含要保存的图像数据。
- plugin (可选) - 指定用于保存图像的插件的字符串。如果未提供 plugin 参数,则该函数将自动尝试不同的插件,从 imageio 开始,直到找到合适的插件。但是,如果文件名 (fname) 具有".tiff"扩展名,则默认情况下将使用 tifffile 插件。
- check_contrast (可选) - 一个布尔值,指示是否检查图像的对比度并打印警告。默认值为 True。
- **plugin_args (可选) - 传递给指定插件的其他关键字参数。
示例 1
以下示例使用imsave()方法将随机数据的数组写入外部图像文件 (.jpg)。
import numpy as np from skimage import io # Generate an image with random numbers image_data = np.random.randint(0, 256, size=(256, 256, 3), dtype=np.uint8) # Save the random image as a JPG file io.imsave('Output/Random_image.jpg', image_data) print("The image was saved successfully.")
输出
The image was saved successfully.
如果我们导航到保存图像的目录,我们将能够观察到保存的"Random_image.jpg"图像,如下所示:

示例 2
以下示例使用 imsave() 方法将图像数组写入 TIFF 文件 (.tiff)。
import numpy as np from skimage import io # Read an image image_data = io.imread('Images/logo-w.png') print("The input image properties:") print('Type:', type(image_data)) print('Shape:', image_data.shape) # Save the image as a tiff file io.imsave('Output/logo.tiff', image_data) print("The image was saved successfully...")
输出
The input image properties: Type: < class 'numpy.ndarray' > Shape: (225, 225, 4) The image was saved successfully...
下图表示保存到图像保存目录中的 "logo.tiff" 文件。

示例 3
以下示例演示了如何使用 imsave() 方法将图像保存为具有低质量的 JPEG 文件 (.jpeg)。
import numpy as np from skimage import io # Read an image image = io.imread('Images/Flower1.jpg') # Save the image as a JPEG file io.imsave('Output/flower.jpeg', image, plugin='pil', quality=10) # Display the image array properties print("The input image properties:") print('Type:', type(image)) print('Shape:', image.shape) print("The image was saved successfully...")
输出
The input image properties: Type: < class 'numpy.ndarray'> Shape: (4000, 6000, 3) The image was saved successfully...
以下图像分别表示输入 (Flower1.jpg) 和保存 (flower.jpeg) 文件在各自目录中的详细信息。
输入文件

保存的文件

广告