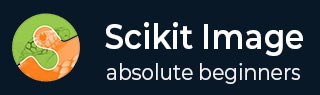
- Scikit Image 教程
- Scikit Image - 简介
- Scikit Image - 图像处理
- Scikit Image - NumPy 图像
- Scikit Image - 图像数据类型
- Scikit Image - 使用插件
- Scikit Image - 图像处理
- Scikit Image - 读取图像
- Scikit Image - 写入图像
- Scikit Image - 显示图像
- Scikit Image - 图像集合
- Scikit Image - 图像堆栈
- Scikit Image - 多张图像
Scikit Image - 使用插件
插件指的是可以增强程序功能的扩展或外部软件组件,通过向其中添加特定功能来提高其功能。
Python Scikit Image 中的插件
Python scikit-image (skimage) 库带有一系列插件,可用于处理图像 IO 操作,例如读取、写入和显示图像。scikit-image 库中可用的插件包括 Matplotlib、PIL(Python Imaging Library)、GDAL、SimpleITK、tifffile、PyFITS 和 ImageIO 等流行库。每个插件都专门用于特定的图像 IO 操作。
scikit-image 库根据需要加载插件,以确保最佳性能并实现有效的资源利用。这意味着只有在明确需要时或设置为默认值时才会加载插件。这种动态加载机制确保仅加载必要的插件,具体取决于特定的图像 I/O 操作。
此外,scikit-image 库还提供了一系列功能工具来处理和操作插件。这些工具允许用户自定义其图像 IO 操作,让我们讨论一下 skimage.io 模块中提供的一些关键插件函数。
列出可用的插件
函数io.find_available_plugins(loaded=False)用于列出 scikit-image (skimage.io) 中可用的插件。它返回一个字典,其中插件名称作为键,公开的函数作为值。以下是此函数的语法:
skimage.io.find_available_plugins(loaded=False)
参数“loaded”采用布尔值。如果将其设置为True,则仅显示已加载的插件。默认情况下,将显示所有插件。
示例 1
以下示例演示了如何使用io.find_available_plugins()函数列出所有可用的插件及其对应的公开函数。
import skimage.io as io # List all available plugins available_plugins = io.find_available_plugins() # Display the plugin names and their exposed functions for plugin_name, exposed_functions in available_plugins.items(): print('Plugin:', plugin_name) print("Exposed Functions:", exposed_functions) print()
输出
Plugin: fits Exposed Functions: ['imread', 'imread_collection'] Plugin: gdal Exposed Functions: ['imread', 'imread_collection'] Plugin: gtk Exposed Functions: ['imshow'] Plugin: imageio Exposed Functions: ['imread', 'imsave', 'imread_collection'] Plugin: imread Exposed Functions: ['imread', 'imsave', 'imread_collection'] Plugin: matplotlib Exposed Functions: ['imshow', 'imread', 'imshow_collection', 'imread_collection'] Plugin: pil Exposed Functions: ['imread', 'imsave', 'imread_collection'] Plugin: qt Exposed Functions: ['imshow', 'imsave', 'imread', 'imread_collection'] Plugin: simpleitk Exposed Functions: ['imread', 'imsave', 'imread_collection'] Plugin: tifffile Exposed Functions: ['imread', 'imsave', 'imread_collection']
示例 2
让我们仅获取有关已加载插件的信息。
from skimage import io # List loaded plugins only available_plugins = io.find_available_plugins(loaded=True) # Display the loaded plugin names and their exposed functions for plugin_name, exposed_functions in available_plugins.items(): print('Plugin:', plugin_name) print("Exposed Functions:", exposed_functions) print()
输出
Plugin: imageio Exposed Functions: ['imread', 'imsave', 'imread_collection'] Plugin: matplotlib Exposed Functions: ['imshow', 'imread', 'imshow_collection', 'imread_collection'] Plugin: tifffile Exposed Functions: ['imread', 'imsave', 'imread_collection']
检索有关特定插件的信息
函数 io.plugin_info(plugin) 用于检索有关特定插件的信息。它返回一个包含有关插件信息的字典,例如描述,并且仅提供可用的函数名称。以下是此函数的语法:
skimage.io.plugin_info(plugin)
参数“plugin”采用字符串,表示要检索信息的插件的名称。
示例 1
以下示例演示了如何使用io.plugin_info()函数检索有关“pil”插件的信息。
from skimage import io # Get information about the 'pil' plugin plugin_info = io.plugin_info('pil') # Print the plugin information print("Description:", plugin_info['description']) print("Available Functions:", plugin_info['provides']) print()
输出
Description: Image reading via the Python Imaging Library Available Functions: imread, imsave
示例 2
在此示例中,我们将获取有关“matplotlib”插件的信息。
from skimage import io # Get information about the 'matplotlib' plugin plugin_info = io.plugin_info('matplotlib') # Print the plugin information print("Description:", plugin_info['description']) print("Available Functions:", plugin_info['provides']) print()
输出
Description: Display or save images using Matplotlib Available Functions: imshow, imread, imshow_collection, _app_show
检索插件顺序
io.plugin_order() 函数用于获取当前首选的插件顺序。以下是此函数的语法:
skimage.io.plugin_order()
该函数返回一个字典,其中函数名称作为键,对应值是按优先级顺序排列的插件列表。
示例
以下是使用 plugin_order() 函数获取当前首选插件顺序的示例。
from skimage import io # Get the currently preferred plugin order order_dict = io.plugin_order() # Print the plugin loading order print("Preferred Plugin Order:") for function_name, plugins in order_dict.items(): print("Function:", function_name) print("Plugins in order of preference:", plugins) print()
输出
Preferred Plugin Order: Function: imread Plugins in order of preference: ['imageio', 'matplotlib'] Function: imsave Plugins in order of preference: ['imageio'] Function: imshow Plugins in order of preference: ['matplotlib'] Function: imread_collection Plugins in order of preference: ['imageio', 'matplotlib'] Function: imshow_collection Plugins in order of preference: ['matplotlib'] Function: _app_show Plugins in order of preference: ['matplotlib']
设置默认插件
io.use_plugin() 函数用于为指定的运算设置默认插件。
如果尚未加载指定的插件,则会加载它。以下是此函数的语法:
skimage.io.use_plugin(name, kind=None)
以下是此函数的参数:
- name - 表示要设置为默认值的插件名称的字符串。
- kind(可选) - 表示为其设置插件的特定函数的字符串。它可以采用以下值之一:“imsave”、“imread”、“imshow”、“imread_collection”、“imshow_collection”。默认情况下,插件设置为所有函数。
示例
以下示例显示了设置默认插件后插件加载顺序的变化。
from skimage import io # Get the currently preferred plugin order order_dict_1 = io.plugin_order() # Print the plugin loading order print("Plugin Order before Setting the default plugin:") for function_name, plugins in order_dict_1.items(): print(function_name) print("Plugin order:", plugins) print() # Set the default plugin for all functions io.use_plugin('qt') # Get the preferred plugin order after setting the default plugin order_dict_2 = io.plugin_order() # Print the plugin loading order print("Plugin Order after setting the default plugin: ") for function_name, plugins in order_dict_2.items(): print(function_name) print("Plugin order:", plugins) print()
输出
Plugin Order before Setting the default plugin: imread Plugin order: ['imageio', 'matplotlib'] imsave Plugin order: ['imageio'] imshow Plugin order: ['matplotlib'] imread_collection Plugin order: ['imageio', 'matplotlib'] imshow_collection Plugin order: ['matplotlib'] _app_show Plugin order: ['matplotlib'] Plugin Order after setting the default plugin: imread Plugin order: ['qt', 'imageio', 'matplotlib'] imsave Plugin order: ['qt', 'imageio'] imshow Plugin order: ['qt', 'matplotlib'] imread_collection Plugin order: ['qt', 'imageio', 'matplotlib'] imshow_collection Plugin order: ['matplotlib'] _app_show Plugin order: ['qt', 'matplotlib']
将插件重置为其默认状态
函数 io.reset_plugins() 用于将插件状态重置为其默认/初始状态。即,不加载任何插件。以下是此函数的语法:
skimage.io.reset_plugins()
示例
以下示例演示了如何使用 io.reset_plugins() 函数将插件状态重置为其默认状态。它在将插件状态重置为默认状态之前和之后检索插件顺序。
from skimage import io # Get the currently preferred plugin order order_dict_1 = io.plugin_order() # Print the plugin loading order print("Plugin Order before Resetting to the default state:") for function_name, plugins in order_dict_1.items(): if function_name == 'imread': print('Function',function_name) print("Plugin order:", plugins) print() # Reset the plugin state to the default io.reset_plugins() # Get the preferred plugin order after Resetting to the default state order_dict_2 = io.plugin_order() # Print the plugin loading order print("Plugin Order after Resetting to the default state: ") for function_name, plugins in order_dict_2.items(): if function_name == 'imread': print('Function',function_name) print("Plugin order:", plugins) print()
输出
Plugin Order before Resetting to the default state: Function imread Plugin order: ['pil', 'imageio', 'matplotlib'] Plugin Order after Resetting to the default state: Function imread Plugin order: ['imageio', 'matplotlib']
特定函数的合适插件
io.call_plugin() 函数用于查找指定函数的合适插件并执行它。以下是此函数的语法:
skimage.io.call_plugin(kind, *args, **kwargs)
以下是此函数的参数:
- kind (str) - 要查找的函数。它可以采用以下值之一:“imshow”、“imsave”、“imread”、“imread_collection”。
- plugin (str, optional) - 要加载的特定插件。如果未提供(默认为 None),则将使用第一个匹配的插件。
- *args, **kwargs - 将传递给插件函数的其他参数和关键字参数。
示例
以下示例演示了如何使用 io.call_plugin() 函数使用图像读取函数的合适插件加载图像文件。
import numpy as np from skimage import io img_array = io.call_plugin('imread', 'Images_/black rose.jpg') print("Image Arary Shape:",img_array.shape)
输出
Image Arary Shape: (2848, 4272, 3)
该函数加载图像文件并将其作为 NumPy 数组返回,该数组分配给 img_array 变量。