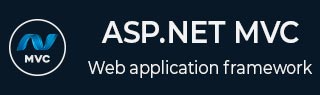
- ASP.NET MVC 教程
- ASP.NET MVC - 首页
- ASP.NET MVC - 概述
- ASP.NET MVC - 模式
- ASP.NET MVC - 环境设置
- ASP.NET MVC - 入门
- ASP.NET MVC - 生命周期
- ASP.NET MVC - 路由
- ASP.NET MVC - 控制器
- ASP.NET MVC - 操作
- ASP.NET MVC - 过滤器
- ASP.NET MVC - 选择器
- ASP.NET MVC - 视图
- ASP.NET MVC - 数据模型
- ASP.NET MVC - 辅助方法
- ASP.NET MVC - 模型绑定
- ASP.NET MVC - 数据库
- ASP.NET MVC - 验证
- ASP.NET MVC - 安全性
- ASP.NET MVC - 缓存
- ASP.NET MVC - Razor
- ASP.NET MVC - 数据注释
- Nuget 包管理
- ASP.NET MVC - Web API
- ASP.NET MVC - 脚手架
- ASP.NET MVC - Bootstrap
- ASP.NET MVC - 单元测试
- ASP.NET MVC - 部署
- ASP.NET MVC - 自托管
- ASP.NET MVC 有用资源
- ASP.NET MVC - 快速指南
- ASP.NET MVC - 有用资源
- ASP.NET MVC - 讨论
ASP.NET MVC - 脚手架
ASP.NET 脚手架是用于 ASP.NET Web 应用程序的代码生成框架。Visual Studio 2013 包含 MVC 和 Web API 项目的预安装代码生成器。当您想要快速添加与数据模型交互的代码时,可以将脚手架添加到您的项目中。使用脚手架可以减少在项目中开发标准数据操作所需的时间。
正如您所看到的,我们已经为 Index、Create、Edit 操作创建了视图,并且还需要更新操作方法。但 ASP.Net MVC 提供了一种更简单的方法来使用脚手架创建所有这些视图和操作方法。
让我们来看一个简单的例子。我们将创建相同的示例,其中包含一个模型类 Employee,但这次我们将使用脚手架。
步骤 1 - 打开 Visual Studio 并点击文件 → 新建 → 项目菜单选项。
将打开一个新的项目对话框。
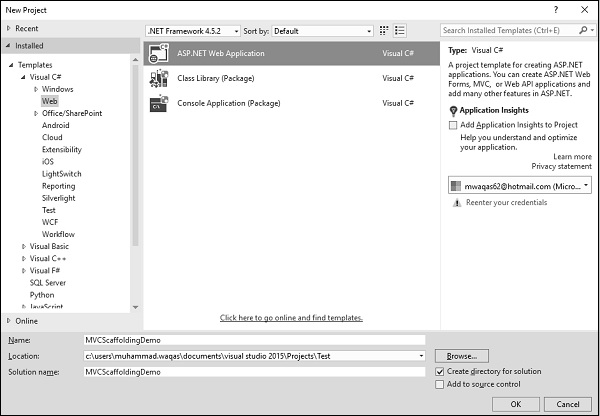
步骤 2 - 从左侧窗格中,选择模板 → Visual C# → Web。
步骤 3 - 在中间窗格中,选择 ASP.NET Web 应用程序。
步骤 4 - 在名称字段中输入项目名称“MVCScaffoldingDemo”,然后点击确定继续。您将看到以下对话框,提示您设置 ASP.NET 项目的初始内容。
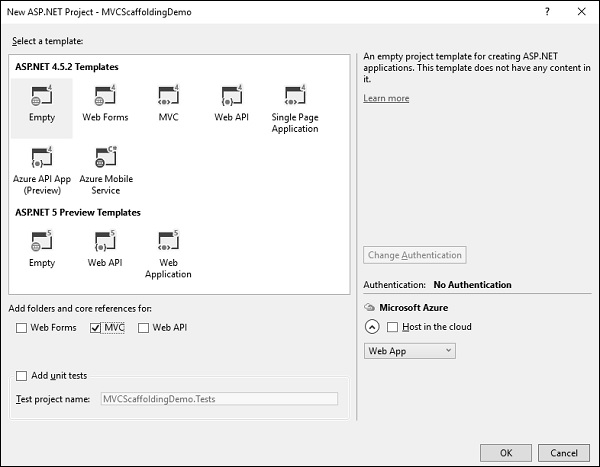
步骤 5 - 为简单起见,选择“空”选项,并在“添加文件夹和核心引用”部分选中 MVC 复选框,然后点击确定。
它将创建一个具有最少预定义内容的基本 MVC 项目。
Visual Studio 创建项目后,您将在解决方案资源管理器窗口中看到许多文件和文件夹。
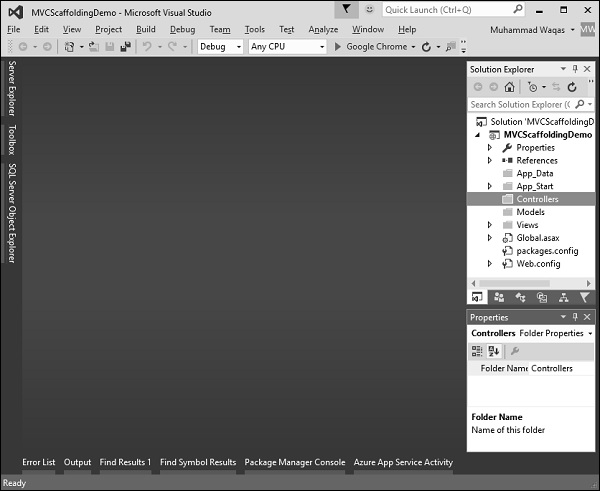
添加 Entity Framework 支持
第一步是安装 Entity Framework。右键点击项目并选择 NuGet 包管理器 → 管理解决方案的 NuGet 包…
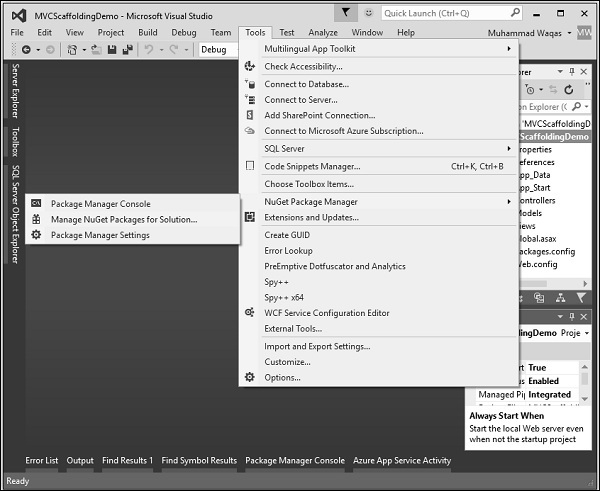
它将打开“NuGet 包管理器”。在搜索框中搜索 Entity framework。
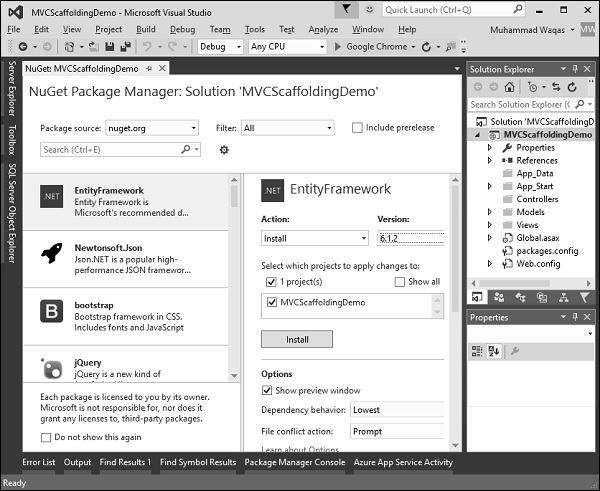
选择 Entity Framework 并点击“安装”按钮。它将打开“预览”对话框。
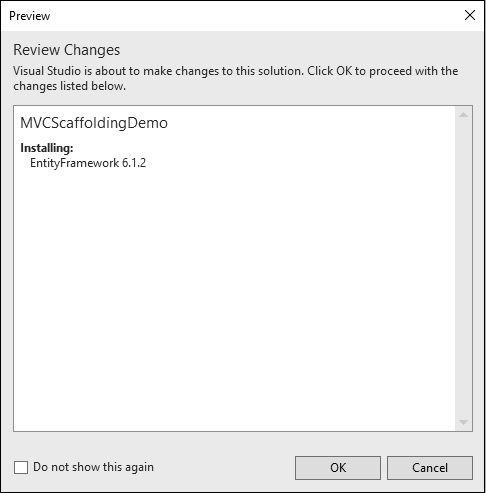
点击确定继续。
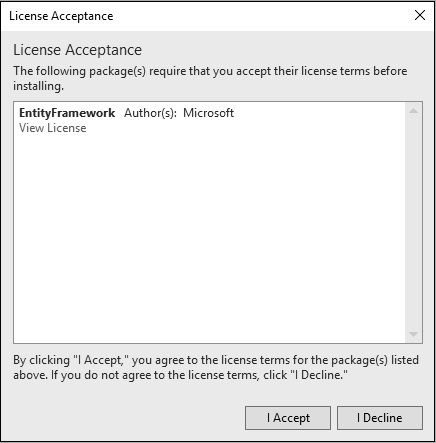
点击“我接受”按钮开始安装。
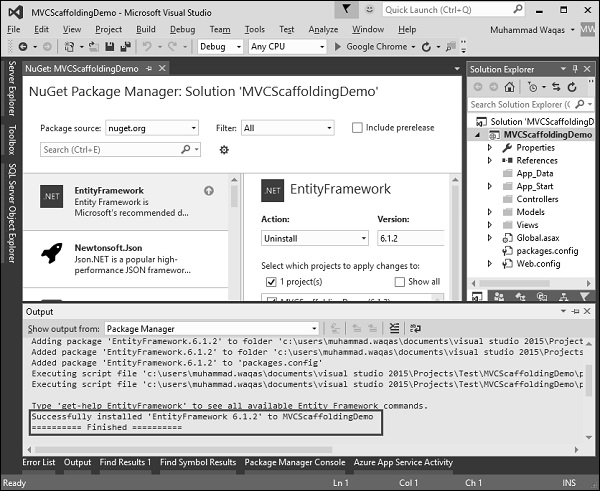
安装 Entity Framework 后,您将在输出窗口中看到消息,如上图所示。
添加模型
要添加模型,请右键点击解决方案资源管理器中的 Models 文件夹,然后选择添加 → 类。您将看到“添加新项”对话框。
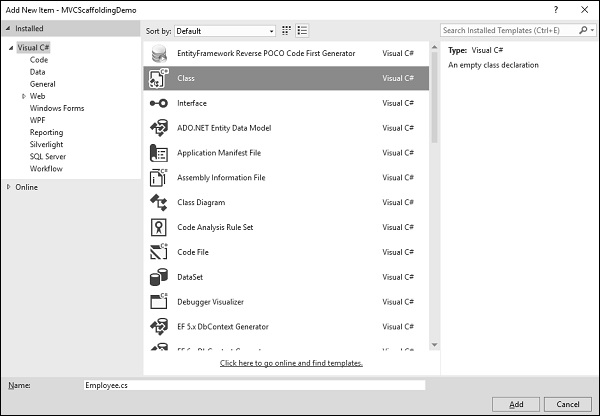
在中间窗格中选择类,并在名称字段中输入 Employee.cs。
使用以下代码向 Employee 类添加一些属性。
using System; namespace MVCScaffoldingDemo.Models { public class Employee{ public int ID { get; set; } public string Name { get; set; } public DateTime JoiningDate { get; set; } public int Age { get; set; } } }
添加 DBContext
我们有一个 Employee 模型,现在我们需要添加另一个类,它将与 Entity Framework 通信以检索和保存数据。以下是 Employee.cs 文件中的完整代码。
using System; using System.Data.Entity; namespace MVCScaffoldingDemo.Models{ public class Employee{ public int ID { get; set; } public string Name { get; set; } public DateTime JoiningDate { get; set; } public int Age { get; set; } } public class EmpDBContext : DbContext{ public DbSet<Employee> Employees { get; set; } } }
如您所见,“EmpDBContext”派生自一个名为“DbContext”的 EF 类。在这个类中,我们有一个名为 DbSet 的属性,它基本上表示您想要查询和保存的实体。
现在让我们构建解决方案,您将在项目成功构建时看到消息。
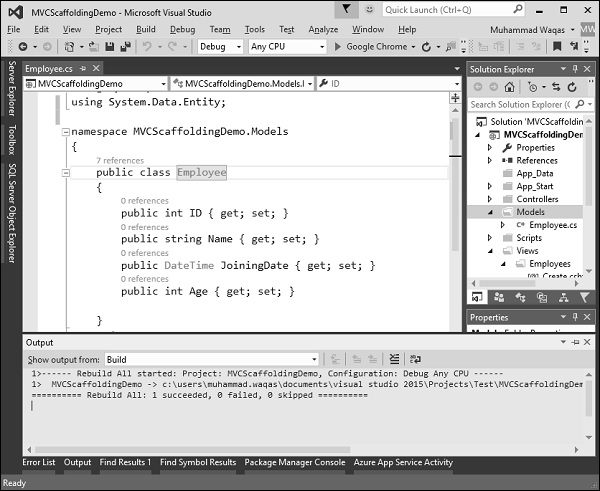
添加脚手架项
要添加脚手架,请右键点击解决方案资源管理器中的 Controllers 文件夹,然后选择添加 → 新建脚手架项。
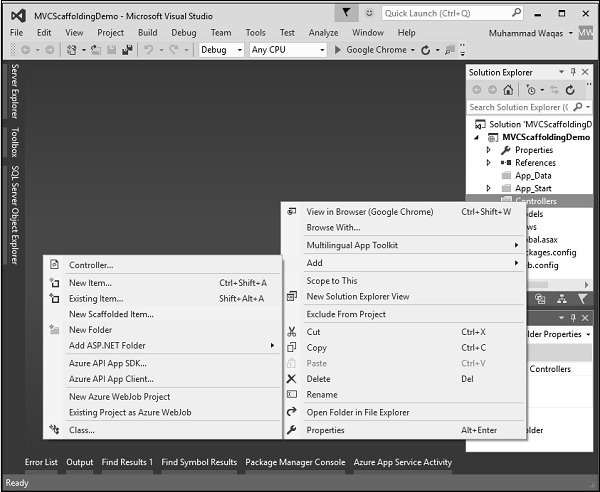
它将显示添加脚手架对话框。
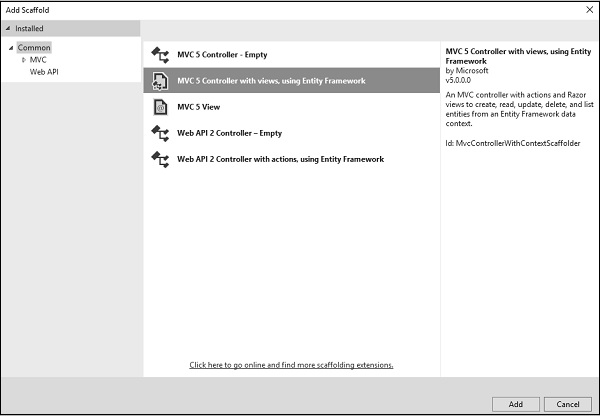
在中间窗格中选择使用 Entity Framework 的 MVC 5 控制器,然后点击“添加”按钮,这将显示添加控制器对话框。
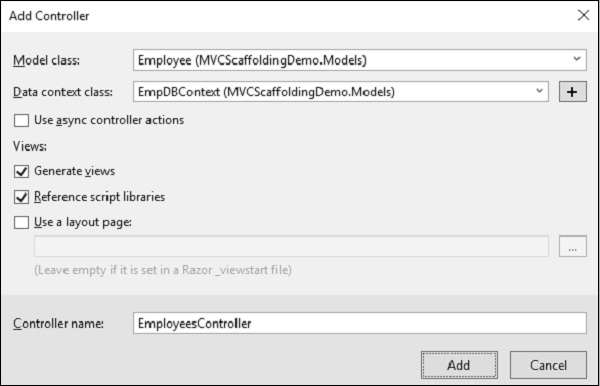
从模型类下拉列表中选择 Employee,从数据上下文类下拉列表中选择 EmpDBContext。您还将看到控制器名称默认为选中状态。
点击“添加”按钮继续,您将在 EmployeesController 中看到以下代码,该代码由 Visual Studio 使用脚手架创建。
using System.Data.Entity; using System.Linq; using System.Net; using System.Web.Mvc; using MVCScaffoldingDemo.Models; namespace MVCScaffoldingDemo.Controllers { public class EmployeesController : Controller{ private EmpDBContext db = new EmpDBContext(); // GET: Employees public ActionResult Index(){ return View(db.Employees.ToList()); } // GET: Employees/Details/5 public ActionResult Details(int? id){ if (id == null){ return new HttpStatusCodeResult(HttpStatusCode.BadRequest); } Employee employee = db.Employees.Find(id); if (employee == null){ return HttpNotFound(); } return View(employee); } // GET: Employees/Create public ActionResult Create(){ return View(); } // POST: Employees/Create // To protect from overposting attacks, please enable the specific properties you want to bind to, for // more details see http://go.microsoft.com/fwlink/?LinkId=317598. [HttpPost] [ValidateAntiForgeryToken] public ActionResult Create([Bind(Include = "ID,Name,JoiningDate,Age")] Employee employee){ if (ModelState.IsValid){ db.Employees.Add(employee); db.SaveChanges(); return RedirectToAction("Index"); } return View(employee); } // GET: Employees/Edit/5 public ActionResult Edit(int? id){ if (id == null){ return new HttpStatusCodeResult(HttpStatusCode.BadRequest); } Employee employee = db.Employees.Find(id); if (employee == null){ return HttpNotFound(); } return View(employee); } // POST: Employees/Edit/5 // To protect from overposting attacks, please enable the specific properties you want to bind to, for // more details see http://go.microsoft.com/fwlink/?LinkId=317598. [HttpPost] [ValidateAntiForgeryToken] public ActionResult Edit([Bind(Include = "ID,Name,JoiningDate,Age")]Employee employee){ if (ModelState.IsValid){ db.Entry(employee).State = EntityState.Modified; db.SaveChanges(); return RedirectToAction("Index"); } return View(employee); } // GET: Employees/Delete/5 public ActionResult Delete(int? id){ if (id == null){ return new HttpStatusCodeResult(HttpStatusCode.BadRequest); } Employee employee = db.Employees.Find(id); if (employee == null){ return HttpNotFound(); } return View(employee); } // POST: Employees/Delete/5 [HttpPost, ActionName("Delete")] [ValidateAntiForgeryToken] public ActionResult DeleteConfirmed(int id){ Employee employee = db.Employees.Find(id); db.Employees.Remove(employee); db.SaveChanges(); return RedirectToAction("Index"); } protected override void Dispose(bool disposing){ if (disposing){ db.Dispose(); } base.Dispose(disposing); } } }
运行您的应用程序并指定以下 URL https://127.0.0.1:59359/employees。您将看到以下输出。
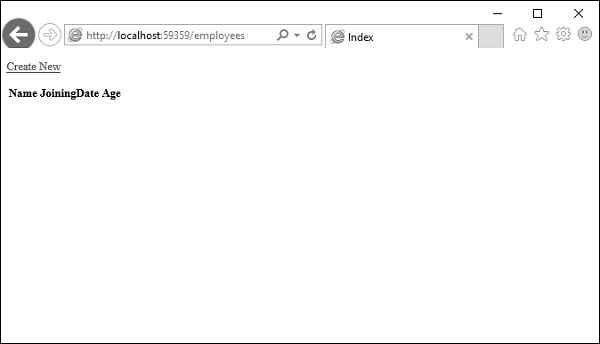
您可以看到 View 中没有数据,因为我们尚未向由 Visual Studio 创建的数据库中添加任何记录。
让我们通过点击“新建”链接从浏览器中添加一条记录,它将显示 Create 视图。
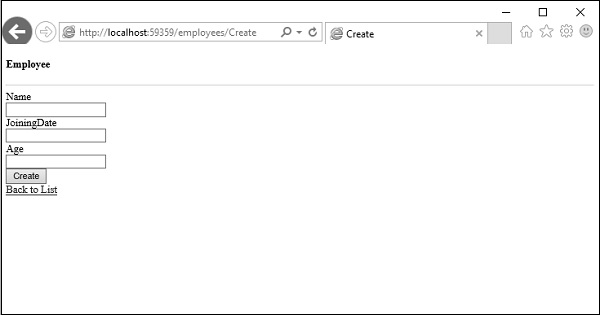
让我们在以下字段中添加一些数据。
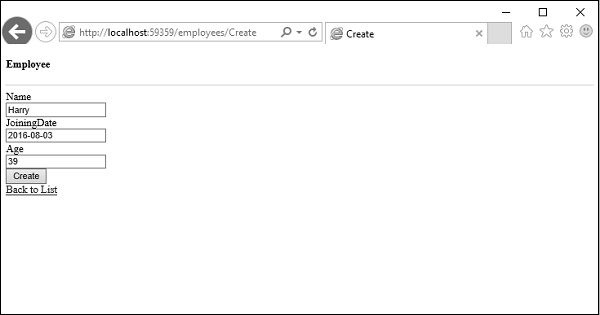
点击“创建”按钮,它将更新 Index 视图。
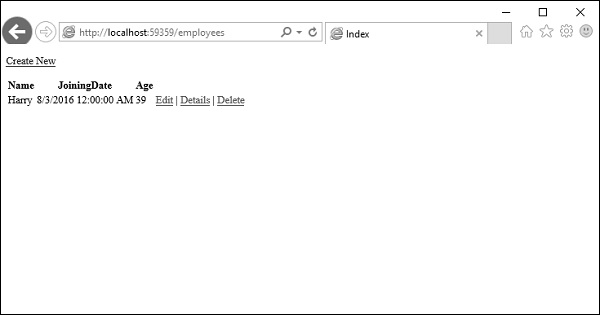
您可以看到新记录也已添加到数据库中。
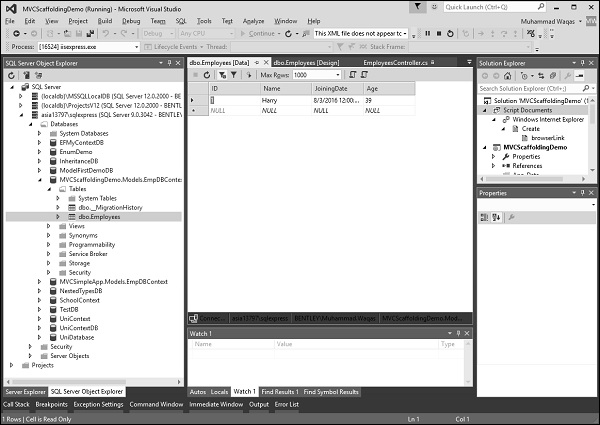
如您所见,我们已经使用脚手架实现了相同的示例,这是一种更简单的方法,可以从您的模型类创建视图和操作方法。