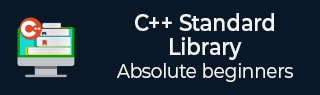
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ 内存::allocate_shared
C++ Memory::allocate_shared 使用 alloc 分配类型为 T 的对象的内存,并使用 args 传递给其构造函数的参数来构造它。通常,此过程使用单个内存分配来分配共享指针控制块以及 T 对象的内存(这是标准中的非绑定性要求)。相比之下,在声明 std::shared_ptr<T> p(new T(Args...)) 中至少进行了两次内存分配,这可能会导致额外的开销。
控制块包含 alloc 的副本,一旦共享和弱引用计数为零,就可以使用它来释放内存。
语法
以下是 C++ Memory::allocate_shared 的语法:
shared_ptr<T> allocate_shared (const Alloc& alloc, Args&&... args);
参数
- args − 这是一个分配器对象。
- alloc − 这是一个零个或多个类型的列表。
示例 1
让我们来看下面的例子,我们在这里使用 allocate_shared 并检索值。
#include <iostream> #include <memory> void result(const std::shared_ptr<int>& i){ (*i)++; } int main(){ std::allocator <int> alloc; std::default_delete <int> del; auto value = std::allocate_shared<int>(alloc,10); result(value); std::cout << *value << std::endl; }
输出
让我们编译并运行上述程序,这将产生以下结果:
11
示例 2
以下是另一种情况,我们将使用 allocate_shared 并检索值。
#include <iostream> #include <memory> int main (){ std::allocator<int> alloc; std::default_delete<int> del; std::shared_ptr<int> Result1 = std::allocate_shared<int> (alloc,11); auto Result2 = std::allocate_shared<int> (alloc,1); std::cout << "*Result1: " << *Result1 << '\n'; std::cout << "*Result2: " << *Result2 << '\n'; return 0; }
输出
运行上述代码后,它将显示如下输出:
*Result1: 11 *Result2: 1
示例 3
考虑以下情况,我们不打算为 allocate_shared 传递任何值,因此它将返回值“0”。
#include <iostream> #include <memory> int main (){ std::allocator<int> alloc; std::default_delete<int> del; std::shared_ptr<int> Result = std::allocate_shared<int> (alloc); std::cout << "*Result: " << *Result << '\n'; return 0; }
输出
代码执行后,将生成如下所示的输出:
*Result: 0
广告