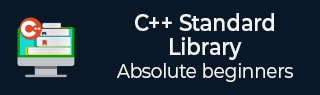
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ 库 - <flat_map>
<flat_map> 头文件是容器库的一部分,提供各种功能作为排序关联容器,用于降低内存使用、快速访问,并存储具有唯一键的键值对。
flat_map 容器充当两个底层容器的包装器,并通过结合有序和连续存储的优势提供单一方法。
包含 <flat_map> 头文件
要在您的 C++ 程序中包含 <flat_map> 头文件,可以使用以下语法。
#include <flat_map>
<flat_map> 头文件的功能
以下是 <flat_map> 头文件中所有函数的列表。
元素访问
元素访问函数提供诸如检索或修改容器中元素的机制。这可以通过使用索引和键来完成。
序号 | 函数及描述 |
---|---|
1 | at
此函数访问具有边界检查的元素。 |
2 | operator[]
此函数访问或插入指定的元素。 |
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
访问元素
在以下示例中,我们将使用 operator[] 来访问或插入元素。
#include <iostream> #include <flat_map> int main() { std::flat_map<int, std::string> myMap; myMap[1] = "One"; myMap[2] = "Two"; std::cout << "Element at key 1: " << myMap[1] << std::endl; return 0; }
输出
如果我们运行上述代码,它将生成以下输出:
Element at key 1: One
迭代器
迭代器提供了一种遍历或操作容器元素的方法。在 flat_map 中,迭代器用于从一个键值对移动到另一个键值对。
序号 | 函数及描述 |
---|---|
1 | begin, cbegin
这些函数返回指向容器开头的迭代器。 |
2 | end, cend
这些函数返回指向容器末尾的迭代器。 |
3 | rbegin, crbegin
这些函数返回指向开头(最后一个元素)的反向迭代器。 |
4 | rend, crend
这些函数返回指向末尾(第一个元素之前)的反向迭代器。 |
检索迭代器
在以下示例中,我们将使用 begin() 获取容器开头的迭代器。
#include <iostream> #include <flat_map> int main() { std::flat_map<int, std::string> myMap = {{1, "One"}, {2, "Two"}}; auto it = myMap.begin(); std::cout << "First element: " << it->t << " -> " << it->second << std::endl; return 0; }
输出
如果我们运行上述代码,它将生成以下输出:
First element: 1 -> One
容量
容量函数用于检查和提供有关容器大小和容量的信息。
序号 | 函数及描述 |
---|---|
1 | empty
此函数检查容器适配器是否为空。 |
2 | size
此函数返回元素的数量。 |
3 | max_size
此函数返回元素的最大可能数量。 |
检查容器是否存在
在以下示例中,我们将使用 empty() 检查容器是否为空。
#include <iostream> #include <flat_map> int main() { std::flat_map<int, std::string> myMap; if (myMap.empty()) { std::cout << "The flat_map is empty." << std::endl; } else { std::cout << "The flat_map is not empty." << std::endl; } return 0; }
输出
如果我们运行上述代码,它将生成以下输出:
The flat_map is empty.
修改器
修改器函数通过插入、替换或删除元素来更改容器的内容。
序号 | 函数及描述 |
---|---|
1 | emplace
此函数在适当位置构造元素。 |
2 | emplace_hint
此函数使用插入位置的提示在适当位置构造元素。 |
3 | try_emplace
如果键不存在,此函数在适当位置插入,如果键存在,则不执行任何操作。 |
4 | insert_or_assign
此函数插入元素或如果键已存在则分配给当前元素。 |
5 | extract
此函数提取底层容器。 |
6 | replace
此函数替换底层容器。 |
7 | erase
此函数擦除元素。 |
8 | erase_if
此函数擦除满足特定条件的所有元素。 |
9 | operator=
此函数将值分配给容器适配器。 |
在适当位置插入元素
在以下示例中,我们将使用 emplace() 在特定位置插入元素。
#include <iostream> #include <flat_map> int main() { std::flat_map<int, std::string> myMap; myMap.emplace(1, "One"); std::cout << "Element emplaced: " << myMap[1] << std::endl; return 0; }
输出
如果我们运行上述代码,它将生成以下输出:
Element emplaced: One
查找
序号 | 函数及描述 |
---|---|
1 | find
此函数查找具有特定键的元素。 |
2 | count
此函数返回与特定键匹配的元素的数量。 |
3 | contains
此函数检查容器是否包含具有特定键的元素。 |
4 | lower_bound
此函数返回指向不小于给定键的第一个元素的迭代器。 |
5 | upper_bound
此函数返回指向大于给定键的第一个元素的迭代器。 |
6 | equal_range
此函数返回与特定键匹配的元素范围。 |
使用键查找元素
在以下示例中,我们将使用 find() 通过键查找元素。
#include <iostream> #include <flat_map> int main() { std::flat_map<int, std::string> myMap = {{1, "One"}, {2, "Two"}}; auto it = myMap.find(1); if (it != myMap.end()) { std::cout << "Found element: " << it->first << " -> " << it->second << std::endl; } else { std::cout << "Element not found." << std::endl; } return 0; }
输出
如果我们运行上述代码,它将生成以下输出:
Found element: 1 -> One
观察者
观察者函数提供有关容器如何运行的信息,特别是关于键比较和访问底层数据结构的信息。
序号 | 函数及描述 |
---|---|
1 | key_comp
此函数用于比较键。 |
2 | value_comp
返回比较 value_type 类型对象中的键的函数。 |
3 | keys
此函数提供对底层值的容器的直接访问。 |
4 | values
此函数提供对底层值的容器的直接访问。 |
比较键
在以下示例中,我们将使用 key_comp() 比较键。
#include <iostream> #include <flat_map> int main() { std::flat_map<int, std::string> myMap = {{1, "One"}, {2, "Two"}}; auto comp = myMap.key_comp(); if (comp(1, 2)) { std::cout << "Key 1 is less than Key 2." << std::endl; } else { std::cout << "Key 1 is not less than Key 2." << std::endl; } return 0; }
输出
如果我们运行上述代码,它将生成以下输出:
Key 1 is less than Key 2.