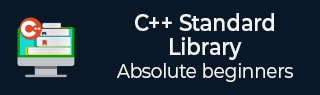
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ 库 - <coroutine>
C++20 中的<coroutine> 库提供了使用协程的基本构建块。协程是允许函数在某些点暂停和恢复的现代特性。它提供了一种有效的异步编程、生成器函数和协作多任务处理机制,从而更容易处理网络、文件 I/O 等任务。
与传统函数不同,协程可以在某些点暂停其执行并在以后恢复。这在需要有效实现非阻塞操作(如文件 I/O 或网络请求)的场景中特别有用。
包含 <coroutine> 头文件
要在 C++ 程序中包含 <coroutine> 头文件,可以使用以下语法。
#include <coroutine>
<coroutine> 头文件的功能
以下是 <coroutine> 头文件中所有函数的列表。
序号 | 函数和描述 |
---|---|
1 | operator=
它赋值 coroutine_handle 对象。 |
2 | operator coroutine_handle<>
它获取一个类型擦除的 coroutine_handle。 |
3 | done
它检查协程是否已完成。 |
4 | operator bool
它检查句柄是否代表一个协程。 |
5 | operator() & resume
它恢复协程的执行。 |
6 | destroy
它销毁一个协程。 |
7 | promise
它访问协程的 promise。 |
8 | address
它导出底层地址。 |
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
生成序列
在下面的示例中,我们将创建一个生成整数序列的协程。
#include <iostream> #include <coroutine> struct x { struct promise_type { int a; int b = 0; x get_return_object() { return x { this }; } std::suspend_always initial_suspend() { return {}; } std::suspend_always final_suspend() noexcept { return {}; } void return_void() {} void unhandled_exception() {} std::suspend_always yield_value(int val) { a = val; return {}; } int getNext() { return b++; } }; promise_type * promise; x(promise_type * p): promise(p) {} int next() { promise -> yield_value(promise -> getNext()); return promise -> a; } }; x generateSequence() { for (int i = 0; i < 4; ++i) { co_yield i; } } int main() { auto sequence = generateSequence(); for (int i = 0; i < 4; ++i) { std::cout << "Next value: " << sequence.next() << '\n'; } return 0; }
输出
以下是上述代码的输出:
Next value: 0 Next value: 1 Next value: 2 Next value: 3
广告