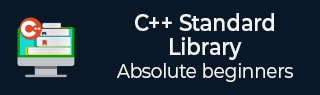
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ 库 - <any>
C++17 中的<any> 头文件提供了一个类型安全的容器,用于存储任何类型的单个值,并允许以安全的方式检索它们。当在编译时无法确定变量的类型,或者函数需要接受多种类型的输入时,它非常有用。
它保存任何类型值的单个实例,并且值的类型存储在内部。它像一个类型擦除容器一样工作,可以保存不同类型的对象,只要这些类型是可复制构造的。但是,除非您执行特定的操作来提取或检查它,否则在运行时无法访问类型信息。
包含 <any> 头文件
要在您的 C++ 程序中包含 <any> 头文件,可以使用以下语法。
#include <any>
<any> 头文件的函数
以下是 <any> 头文件中所有函数的列表。
序号 | 函数和描述 |
---|---|
1 | operator=
赋值任何对象。 |
2 | emplace
更改包含的对象,直接构造新对象。 |
3 | reset
销毁包含的对象。 |
4 | swap
交换两个 any 对象。 |
5 | has_value
检查对象是否包含值。 |
6 | type
返回包含值的 typeid。 |
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
确定值的类型
在下面的示例中,我们将使用 x 最初保存整数,然后重新赋值为保存字符串值,然后使用 type() 函数检查存储值的类型。
#include <iostream> #include <any> #include <string> int main() { std::any x = 1; if (x.type() == typeid(int)) { std::cout << "Integer value: " << std::any_cast < int > (x) << std::endl; } x = std::string("Welcome"); if (x.type() == typeid(std::string)) { std::cout << "String value: " << std::any_cast < std::string > (x) << std::endl; } return 0; }
输出
以下是上述代码的输出:
Integer value: 1 String value: Welcome
广告