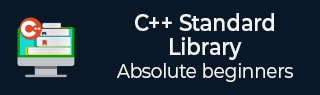
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ 库 - <semaphore>
在 C++20 中,<semaphore> 头文件用于通过多个线程控制对共享资源的访问。它用于限制同时访问某个部分或共享资源的线程数量的场景。
<semaphore> 可以处于两种状态:二进制信号量或计数信号量。其中,binary_semaphore 允许单个线程一次访问一个资源,而 counting_semaphore 允许最多一定数量的线程访问。当计数达到零时,其他线程会被阻塞,直到计数再次大于零。
counting_semaphore 持有一个整数值,而 binary_semaphore 仅限于 0 或 1。
包含 <semaphore> 头文件
要在 C++ 程序中包含 <semaphore> 头文件,可以使用以下语法。
#include <semaphore>
<semaphore> 头文件的函数
以下是 <semaphore> 头文件中所有函数的列表。
序号 | 函数及描述 |
---|---|
1 | release
它递增内部计数器并解除阻塞获取者。 |
2 | acquire
它递减内部计数器或阻塞直到可以递减。 |
3 | try_acquire
它尝试递减内部计数器而不阻塞。 |
4 | try_acquire_for
它尝试递减内部计数器,阻塞最多持续时间。 |
5 | try_acquire_until
它尝试递减内部计数器,阻塞直到某个时间点。 |
5 | max
它返回内部计数器的最大可能值。 |
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
控制访问
在以下示例中,我们将使用计数信号量将对共享资源的访问限制为一次最多两个线程。
#include <iostream> #include <semaphore> #include <thread> std::counting_semaphore < 2 > sem(2); void a(int b) { sem.acquire(); std::cout << "A" << b << " Accessing Resource.\n"; std::this_thread::sleep_for(std::chrono::seconds(1)); std::cout << "A" << b << " Releasing Resource.\n"; sem.release(); } int main() { std::thread x1(a, 1); std::thread x2(a, 2); std::thread x3(a, 3); x1.join(); x2.join(); x3.join(); return 0; }
输出
以上代码的输出如下:
A1 Accessing Resource. A2 Accessing Resource. A1 Releasing Resource. A3 Accessing Resource. A2 Releasing Resource. A3 Releasing Resource.
广告