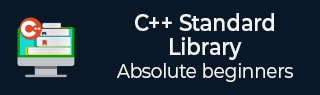
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ 库 - <stop_token>
C++20 中的<stop_token> 头文件引入了一种取消线程和异步操作的机制。它与 `std::stop_source` 配合使用,`std::stop_source` 负责生成停止请求。
这些用于实现响应式系统,该系统可以停止操作,而无需强制终止或复杂的信号机制。
包含 <stop_token> 头文件
要在 C++ 程序中包含 <stop_token> 头文件,可以使用以下语法。
#include <stop_token>
<stop_token> 头文件的函数
以下是 <stop_token> 头文件中所有函数的列表。
序号 | 函数及描述 |
---|---|
1 | operator=
它赋值 `stop_token` 对象。 |
2 | swap
它交换两个 `stop_token` 对象。 |
3 | stop_requested
它检查关联的停止状态是否已请求停止。 |
4 | stop_possible
它检查关联的停止状态是否可以请求停止。 |
5 | get_token
它返回关联停止状态的 `stop_token`。 |
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
使用停止令牌的多线程
在下面的示例中,我们将使用 `std::stop_source` 来控制多个线程。
#include <iostream> #include <thread> #include <stop_token> #include <chrono> void a(int id, std::stop_token b) { while (!b.stop_requested()) { std::cout << "A " << id << " Is Working.." << std::endl; std::this_thread::sleep_for(std::chrono::milliseconds(600)); } std::cout << "A " << id << " Is Cancelled.." << std::endl; } int main() { std::stop_source x; std::thread x1(a, 1, x.get_token()); std::thread x2(a, 2, x.get_token()); std::this_thread::sleep_for(std::chrono::seconds(2)); x.request_stop(); x1.join(); x2.join(); return 0; }
输出
以上代码的输出如下:
A 2 Is Working.. A 1 Is Working.. A 2 Is Working.. A 1 Is Working.. A 2 Is Working.. A 1 Is Working.. A 2 Is Working.. A 1 Is Working.. A 2 Is Cancelled.. A 1 Is Cancelled..
广告