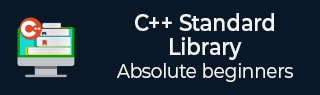
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ istream::operator>>() 函数
C++ 的std::istream::operator>>()函数是一个提取运算符,用于将格式化数据从字符串流对象读取到变量中。它的工作方式类似于与std::cin一起使用的>>运算符,但它从字符串缓冲区中提取数据。
此函数有 3 个多态变体:使用算术类型或流缓冲区或操纵器(您可以在下面找到所有变体的语法)。
语法
以下是 std::istream::operator>>() 函数的语法。
istream& operator>> (bool& val); istream& operator>> (short& val); istream& operator>> (unsigned short& val); istream& operator>> (int& val); istream& operator>> (unsigned int& val); istream& operator>> (long& val); istream& operator>> (unsigned long& val); istream& operator>> (long long& val); istream& operator>> (unsigned long long& val); istream& operator>> (float& val); istream& operator>> (double& val); istream& operator>> (long double& val); istream& operator>> (void*& val); or istream& operator>> (streambuf* sb ); or istream& operator>> (istream& (*pf)(istream&)); istream& operator>> (ios& (*pf)(ios&)); istream& operator>> (ios_base& (*pf)(ios_base&));
参数
- val - 它指示存储提取的字符所表示的值的对象。
- sb - 它指示指向 basic_streambuf 对象的指针,字符将复制到其控制的输出序列上。
- pf - 它指示一个函数,该函数获取并返回一个流对象。
返回值
此函数返回 basic_istream 对象(*this)。
异常
如果抛出异常,则对象处于有效状态。
数据竞争
修改 val 或 sb 指向的对象。
示例
让我们看下面的例子,我们将读取单个整数。
#include <iostream> #include <sstream> #include <string> int main() { std::string a = "11"; std::stringstream b(a); int x; b >> x; std::cout << "Result : " << x << std::endl; return 0; }
输出
以上代码的输出如下:
Result : 11
示例
考虑下面的例子,我们将读取多个数据类型。
#include <iostream> #include <sstream> #include <string> int main() { std::string a = "11 2.3 TutorialsPoint"; std::stringstream b(a); int x; float y; std::string z; b >> x >> y >> z; std::cout << "x : " << x << ", y : " << y << ", z : " << z << std::endl; return 0; }
输出
以下是以上代码的输出:
x : 11, y : 2.3, z : TutorialsPoint
示例
在下面的示例中,我们将读取整数列表。
#include <iostream> #include <sstream> #include <string> #include <vector> int main() { std::string x = "1 2 3 4 5"; std::stringstream y(x); std::vector<int> a; int b; while (y >> b) { a.push_back(b); } std::cout << "Result :"; for (int n : a) { std::cout << " " << n; } std::cout << std::endl; return 0; }
输出
如果我们运行以上代码,它将生成以下输出:
Result : 1 2 3 4 5
istream.htm
广告