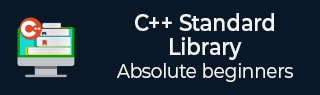
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ Deque::at() 函数
C++ 的std::deque::at() 函数用于根据元素的位置索引提供对元素的访问,允许进行读写操作。当需要进行精确的边界检查时,它对于访问 deque 中的元素非常有用。
与 operator[] 不同,at() 函数会验证索引是否在边界范围内,如果索引无效则抛出超出范围异常。
语法
以下是 std::deque::at() 函数的语法。
reference at (size_type n); const_reference at (size_type n) const;
参数
- n - 表示容器中元素的位置。
返回值
此函数返回容器中指定位置处的元素。
异常
如果 n 无效,则抛出超出范围异常。
时间复杂度
此函数的时间复杂度为常数,即 O(1)。
示例
让我们看下面的示例,我们将演示 at() 函数的基本用法。
#include <iostream> #include <deque> int main() { std::deque<char> a = {'A', 'B', 'C', 'D'}; char element = a.at(2); std::cout << "Element at given index : " << element << std::endl; return 0; }
输出
以上代码的输出如下:
Element at given index : C
示例
考虑以下示例,我们将修改元素。
#include <iostream> #include <deque> int main() { std::deque<int> a = {1,22,3,4444}; a.at(2) = 333; for (int elem : a) { std::cout << elem << " "; } std::cout << std::endl; return 0; }
输出
以下是以上代码的输出:
1 22 333 4444
示例
在以下示例中,我们将访问超出范围的元素并观察输出。
#include <iostream> #include <deque> int main() { std::deque<char> a = {'A', 'B', 'C', 'D'}; try { char element = a.at(6); std::cout << "Element at given index : " << element << std::endl; } catch (const std::out_of_range& oor) { std::cerr << "Out of Range error: " << oor.what() << std::endl; } return 0; }
输出
如果我们运行以上代码,它将生成以下输出:
Out of Range error: deque::_M_range_check: __n (which is 6)>= this->size() (which is 4)
deque.htm
广告