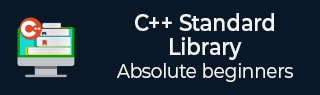
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ Deque::cbegin() 函数
C++ 的 std::deque::cbegin() 函数用于返回指向第一个元素的常量迭代器,允许只读访问而不会修改容器。这对于迭代 deque 中的元素同时确保它们保持不变非常有用。它提供了一种安全且不可修改的方式来访问 deque 开头的元素。
语法
以下是 std::deque::cbegin() 函数的语法。
const_iterator cbegin() const noexcept;
参数
它不接受任何参数。
返回值
此函数返回指向序列开头的 const_iterator。
异常
此函数从不抛出异常。
时间复杂度
此函数的时间复杂度为常数,即 O(1)
示例
在下面的示例中,我们将考虑 cbegin() 函数的基本用法。
#include <iostream> #include <deque> int main() { std::deque<char> x = {'A', 'B', 'C', 'D'}; auto a = x.cbegin(); while (a != x.cend()) { std::cout << *a << " "; ++a; } return 0; }
输出
以上代码的输出如下:
A B C D
示例
考虑以下示例,我们将使用 cbegin() 函数访问第一个元素
#include <iostream> #include <deque> int main() { std::deque<std::string> a = {"TutorialsPoint", "Tutorix", "TP"}; std::cout << " " << *a.cbegin() << std::endl; return 0; }
输出
以上代码的输出如下:
TutorialsPoint
示例
在下面的示例中,我们将使用 cbegin() 函数获取常量迭代器并将第一个元素与值进行比较。
#include <iostream> #include <deque> int main() { std::deque<char> a = {'A', 'B', 'C', 'D'}; auto x = a.cbegin(); if (*x == 'A') { std::cout << "First Element is equal to given value." << std::endl; } else { std::cout << "First Element is not equal to given value." << std::endl; } return 0; }
输出
如果运行以上代码,它将生成以下输出:
First Element is equal to given value.
deque.htm
广告