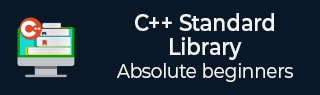
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ Deque::operator>=() 函数
C++ 的std::deque::operator>=()函数用于按字典顺序比较两个双端队列。比较持续进行,直到发现一个双端队列大于另一个双端队列。如果一个双端队列的元素大于或等于另一个双端队列的对应元素,则返回true,否则返回false。
语法
以下是std::deque::的语法。
bool operator>= (const deque<T,Alloc>& lhs, const deque<T,Alloc>& rhs);
参数
- lhs, rhs − 表示双端队列容器。
返回值
如果条件成立,则返回true,否则返回false。
异常
此函数从不抛出异常。
时间复杂度
此函数的时间复杂度为线性,即 O(n)
示例
在下面的示例中,我们将考虑operator>=()函数的基本用法。
#include <iostream> #include <deque> int main() { std::deque<int> a = {10,12,23}; std::deque<int> b = {01,12,23}; if (a >= b) { std::cout << "a is greater than or equal to b." << std::endl; } else { std::cout << "a is not greater than or equal to b." << std::endl; } return 0; }
输出
以上代码的输出如下:
a is greater than or equal to b.
示例
考虑以下示例,我们将比较不同大小的双端队列。
#include <iostream> #include <deque> int main() { std::deque<int> a = {1, 2}; std::deque<int> b = {1, 2, 4}; if (a >= b) { std::cout << "a is greater than or equal to b." << std::endl; } else { std::cout << "a is not greater than or equal to b." << std::endl; } return 0; }
输出
以上代码的输出如下:
a is not greater than or equal to b.
示例
让我们看下面的例子,我们将用字符串填充双端队列并进行比较。
#include <iostream> #include <deque> #include <string> int main() { std::deque<std::string> a = {"Ducati", "Cheron"}; std::deque<std::string> b = {"Aston", "Lexus"}; if (a >= b) { std::cout << "a is greater than or equal to b." << std::endl; } else { std::cout << "a is not greater than or equal to b." << std::endl; } return 0; }
输出
如果我们运行以上代码,它将生成以下输出:
a is greater than or equal to b.
deque.htm
广告