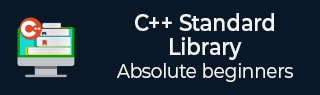
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ Deque::shrink_to_fit() 函数
C++ 的std::deque::shrink_to_fit() 函数用于将 deque 的容量减少到适合其大小,释放未使用的内存。此函数是非约束性的,这意味着它充当对实现的请求,并且可能并不总是导致容量减少。它对容器的大小或元素没有影响。
语法
以下是 std::deque::shrink_to_fit() 函数的语法。
void shrink_to_fit();
参数
它不接受任何参数。
返回值
此函数不返回任何内容。
异常
此函数从不抛出异常。
时间复杂度
此函数的时间复杂度为常数,即 O(1)。
示例
在以下示例中,我们将考虑 shrink_to_fit() 函数的基本用法。
#include <iostream> #include <deque> int main() { std::deque<int> a; for (int x = 0; x < 10; ++x) { a.push_back(x); } std::cout << "Deque size before shrink_to_fit(): " << a.size() << std::endl; a.shrink_to_fit(); std::cout << "Deque size after shrink_to_fit(): " << a.size() << std::endl; return 0; }
输出
以上代码的输出如下:
Deque size before shrink_to_fit(): 10 Deque size after shrink_to_fit(): 10
示例
考虑以下示例,我们将对 deque 使用 clear 和 shrink 操作。
#include <iostream> #include <deque> int main() { std::deque<int> a; for (int x = 0; x < 5; ++x) { a.push_back(x); } a.clear(); a.shrink_to_fit(); std::cout << "Deque size after clear and shrink: " << a.size() << std::endl; return 0; }
输出
以下是以上代码的输出:
Deque size after clear and shrink: 0
示例
在以下示例中,我们将使用调整大小后的 shrink 操作。
#include <iostream> #include <deque> int main() { std::deque<int> a; for (int x = 0; x < 10; ++x) { a.push_back(x); } a.resize(5); a.shrink_to_fit(); std::cout << "Deque capacity after resizing and shrink: " << a.size() << std::endl; return 0; }
输出
如果我们运行以上代码,它将生成以下输出:
Deque capacity after resizing and shrink: 5
deque.htm
广告