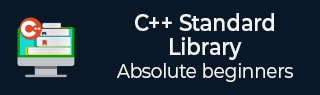
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ 内存::dynamic_pointer_cast
一种称为强制转换的操作符将数据从一种类型转换为另一种类型。C++ 中的动态强制转换主要用于运行时安全的向下转换。动态转换要起作用,基类中需要有一个虚函数。只有多态基类才能使用动态转换,因为它们使用这些数据来确定安全的向下转换。
语法
以下是 C++ Memory::dynamic_pointer_cast 的语法:
shared_ptr<T> dynamic_pointer_cast (const shared_ptr<U>& sp) noexcept;
参数
sp − 这是一个共享指针。
示例 1
让我们来看下面的例子,我们将执行 dynamic_pointer_cast 并获取输出。
#include <iostream> #include <exception> using namespace std; class Base { virtual void dummy() {} }; class Derived: public Base { int a; }; int main (){ try { Base * pba = new Derived; Base * pbb = new Base; Derived * pd; pd = dynamic_cast<Derived*>(pba); if (pd==0) cout << "Welcome \n"; pd = dynamic_cast<Derived*>(pbb); if (pd==0) cout << "TutorialsPoint\n"; } catch (exception& e) { cout << "Exception: " << e.what(); } return 0; }
输出
让我们编译并运行上面的程序,这将产生以下结果:
TutorialsPoint
示例 2
考虑下面的例子,其中 dynamic_pointer_cast 在类层次结构中向下/横向转换。
#include <iostream> #include <memory> class Base { public: int a; virtual void f() const { std::cout << "Welcome To\n"; } virtual ~Base() {} }; class Derived : public Base { public: void f() const override { std::cout << "TutoriaslPoint\n"; } ~Derived() {} }; int main(){ auto basePtr = std::make_shared<Base>(); std::cout << "Base pointer: "; basePtr->f(); auto derivedPtr = std::make_shared<Derived>(); std::cout << "Derived pointer: "; derivedPtr->f(); basePtr = std::static_pointer_cast<Base>(derivedPtr); std::cout << "Base pointer to derived : "; basePtr->f(); auto downcastedPtr = std::dynamic_pointer_cast<Derived>(basePtr); if (downcastedPtr) { std::cout << "Downcasted pointer : "; downcastedPtr->f(); } }
输出
运行上述代码后,它将显示如下输出:
Base pointer: Welcome To Derived pointer: TutoriaslPoint Base pointer to derived : TutoriaslPoint Downcasted pointer : TutoriaslPoint
示例 3
在下面的例子中,我们将使用 dynamic_pointer_cast 并静态和动态地获取输出。
#include <iostream> #include <memory> struct A { static const char* static_type; const char* dynamic_type; A(){ dynamic_type = static_type; } }; struct B: A { static const char* static_type; B(){ dynamic_type = static_type; } }; const char* A::static_type = "Hello"; const char* B::static_type = "Welcome"; int main (){ std::shared_ptr<A> Result; std::shared_ptr<B> bar; bar = std::make_shared<B>(); Result = std::dynamic_pointer_cast<A>(bar); std::cout << "Result in static type: " << Result->static_type << '\n'; std::cout << "Result in dynamic type: " << Result->dynamic_type << '\n'; return 0; }
输出
代码执行后,将生成如下所示的输出:
Result in static type: Hello Result in dynamic type: Welcome
广告