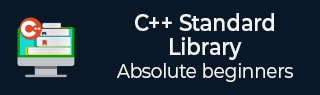
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ Atomic::fetch_xor() 函数
C++ 的std::atomic::fetch_xor() 函数是一个原子操作,用于在原子变量和指定值之间执行原子按位异或运算。此函数确保原子变量的线程安全操作,防止数据竞争。
此函数有两种变体:一种具有指定的内存顺序,控制内存操作如何同步,另一种是默认内存顺序。
语法
以下是 std::atomic::fetch_xor() 函数的语法。
T fetch_xor (T val, memory_order sync = memory_order_seq_cst) volatile noexcept; T fetch_xor (T val, memory_order sync = memory_order_seq_cst) noexcept;
参数
- val - 表示要应用的值。
- sync - 表示操作的同步模式。
返回值
此函数返回调用之前的包含值。
异常
此函数从不抛出异常。
示例
在以下示例中,我们将考虑 fetch_xor() 函数的基本用法。
#include <iostream> #include <atomic> int main() { std::atomic<int> x(0b1111); int result = x.fetch_xor(0b1010); std::cout << "Initial Value: " << result << std::endl; std::cout << "Final Value : " << x.load() << std::endl; return 0; }
输出
以上代码的输出如下:
Initial Value: 15 Final Value : 5
示例
考虑以下示例,我们将使用循环来翻转原子变量的特定位。在每次迭代中都会调用 fetch_xor() 函数。
#include <iostream> #include <atomic> int main() { std::atomic<int> x(0); for (int i = 0; i < 4; ++i) { int a = x.fetch_xor(1 << i); std::cout << "Result after " << i << " iteration : " << a << std::endl; } std::cout << "Final value : " << x.load() << std::endl; return 0; }
输出
以下是以上代码的输出:
Result after 0 iteration : 0 Result after 1 iteration : 1 Result after 2 iteration : 3 Result after 3 iteration : 7 Final value : 15
示例
让我们看看以下示例,我们将在线程环境中使用 fetch_xor()。
#include <iostream> #include <atomic> #include <thread> #include <vector> std::atomic<int> x(0b10101); void a(int b) { x.fetch_xor(b); } int main() { std::vector<std::thread> x1; for (int i = 0; i < 4; ++i) { x1.emplace_back(a, 1 << i); } for (auto& t : x1) { t.join(); } std::cout << "Result : " << x.load() << std::endl; return 0; }
输出
如果我们运行以上代码,它将生成以下输出:
Result : 26
atomic.htm
广告