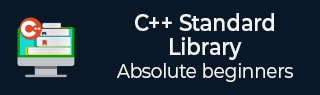
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ List::back() 函数
C++ 的 std::list::back() 函数用于检索列表中最后一个元素的引用。
在 C++ 中,引用是一个值,它使程序能够间接访问特定数据,例如变量或记录。如果当前容器(或列表)非空,则表达式 list.back() 等效于 *std::prev(list.end())。如果当前列表(int 类型)为空,则 back() 函数返回零。
对空列表调用 back() 函数会导致未定义的行为。
语法
以下是 C++ std::list::back() 函数的语法:
reference back();
参数
- 它不接受任何参数。
返回值
此函数返回列表中最后一个元素的引用。
示例 1
在下面的程序中,我们使用 std::list::back() 函数显示当前列表 {1,2,3,4,5} 的最后一个元素。
#include<iostream> #include<list> using namespace std; int main() { //create an integer list list<int> l = {1, 2, 3, 4, 5}; cout<<"The list elements are: "; for(int lst : l){ cout<<lst<<" "; } cout<<"\nLast element of the list is: "<<l.back()<< endl; return 0; }
输出
执行上述程序后,将产生以下输出:
The list elements are: 1 2 3 4 5 Last element of the list is: 5
示例 2
以下是 std::list::back() 函数的另一个示例,这里我们创建一个名为 char_list 的列表(char 类型),其值为 {'a','b','c','d','e'}。然后,使用 back() 函数,我们尝试检索当前列表的最后一个元素。
#include <iostream> #include <list> using namespace std; int main() { //create a character list list<char>char_list = {'a','b','c','d','e'}; cout<<"The list elements are: "; for(char lst : char_list){ cout<<lst<<" "; } cout<<"\nLast element of the list is: "<<char_list.back()<< endl; return 0; }
输出
以下是上述程序的输出:
The list elements are: a b c d e Last element of the list is: e
示例 3
如果当前列表是字符串类型。
以下程序显示当前列表(字符串类型){'Aman', 'Rohit', 'Rahul', 'Reeta'} 的最后一个元素。
#include <iostream> #include <list> #include<string> using namespace std; int main() { list<string> Name; Name.push_back("Aman"); Name.push_back("Rohit"); Name.push_back("Rahul"); Name.push_back("Reeta"); cout<<"The list elements are: "; for(string s: Name){ cout<<s<<" "; } string name = Name.front(); cout <<"\nThe first element in list is: "<<name; return 0; }
输出
上述程序生成的输出如下:
The list elements are: Aman Rohit Rahul Reeta The first element in list is: Reeta
示例 4
如果列表(int 类型)为空,此函数返回零 (0)。
在这个程序中,我们创建一个空列表(int 类型)。然后,使用 std::list::back() 函数,我们尝试获取当前列表(空)的最后一个元素。
#include <iostream> #include <list> using namespace std; int main() { //create a character list list<int> l = {}; cout<<"The list elements are: "; for(int lst : l){ cout<<lst<<" "; } cout<<"\nLast element of the list is: "<<l.back()<<endl; return 0; }
输出
上述程序生成以下输出:
The list elements are: Last element of the list is: 0
示例 5
在下面的示例中,我们创建一个值为 {'A', 'B','C', 'D'} 的列表(char 类型)。然后,我们声明一个名为 result 的 int 变量,它存储 list.back() 函数的值,当我们打印它的值时,它显示此列表最后一个元素的 ASCII 值。
#include <iostream> #include <list> using namespace std; int main() { list<char> char_list = {'A', 'B', 'C', 'D'}; cout<<"The list elements are: "; for(char lst: char_list){ cout<<lst<<" "; } //declare an int variable int result; result = char_list.back(); cout<<"\nLast element of the list is: "<<char_list.back()<<endl; cout<<"The ASCII value of "<<char_list.back()<<" is: "<<result; }
输出
执行上述程序后,它会产生以下输出:
The list elements are: A B C D Last element of the list is: D The ASCII value of D is: 68