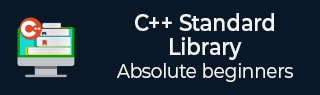
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ List::cbegin() 函数
C++ 的std::list::cbegin() 函数用于获取指向列表第一个元素的常量迭代器。cbegin() 函数类似于 C++ std::list 中的 begin() 函数。其中,begin() 函数用于获取指向列表第一个元素的迭代器,而 cbegin() 函数返回指向列表第一个元素的常量迭代器。
迭代器和常量迭代器的区别在于,常量迭代器不能用于修改其指向的内容。
语法
以下是 C++ std::list::cbegin() 函数的语法:
const_iterator cbegin() const;
参数
- 它不接受任何参数。
返回值
此函数返回一个常量迭代器。
示例 1
在以下程序中,我们使用 C++ std::list::cbegin() 函数来获取指向当前列表 {10, 20, 30, 40, 50} 的第一个(或开头)元素的常量迭代器。
#include<iostream> #include<list> using namespace std; int main() { //create a list list<int> num_list = {10, 20, 30, 40, 50}; cout<<"List elements are: "; for(int n : num_list) { cout<<n<<" "; } auto ri = num_list.cbegin(); cout<<"\nThe constant iterator of the first element: "; cout<<*ri; }
输出
以下是上述程序的输出:
List elements are: 10 20 30 40 50 The constant iterator of the first element: 10
示例 2
除了 int 类型的列表元素外,您还可以检索任何其他类型的列表元素(如 char 和 string 列表内容)的迭代器。
以下是 C++ std::list::cbegin() 函数的另一个示例。在这里,我们创建一个名为 vowels 的列表(类型为 char),其值为 {'a', 'e', 'i', 'o', 'u'}。然后使用 cbegin() 函数,我们尝试获取指向当前列表第一个元素的常量迭代器。
#include<iostream> #include<list> using namespace std; int main() { //create a list list<char> vowels = {'a', 'e', 'i', 'o', 'u'}; cout<<"List elements are: "; for(char v : vowels) { cout<<v<<" "; } auto ri = vowels.cbegin(); cout<<"\nThe constant iterator of the first element: "; cout<<*ri; }
输出
这将生成以下输出:
List elements are: a e i o u The constant iterator of the first element: a
示例 3
如果列表类型是字符串。
在此示例中,我们创建一个名为 fname 的列表(类型为字符串),其值为 {"Rohan", "Reema", "Geeta", "Seema"}。然后,使用 cbegin() 函数,我们尝试获取指向当前列表第一个元素的常量迭代器。
#include<iostream> #include<list> using namespace std; int main() { //create a list list<string> names = {"Rohan", "Reema", "Geeta", "Seema"}; cout<<"List elements are: "; for(string s : names) { cout<<s<<" "; } auto ri = names.cbegin(); cout<<"\nThe constant iterator of first element: "; cout<<*ri; }
输出
上述程序产生以下输出:
List elements are: Rohan Reema Geeta Seema The constant iterator of the first element: Rohan
示例 4
使用 for 循环以及 cbegin() 函数来检索容器元素的迭代器。
在此示例中,我们使用 cbegin() 函数以及 for 循环来遍历当前容器 {1, 2, 3, ,4 ,5} 并从第一个元素开始检索容器中所有元素的常量迭代器。
#include<iostream> #include<list> using namespace std; int main() { //create a list list<int> num_list = {1, 2, 3, 4 ,5}; cout<<"List elements are: "; for(int n : num_list) { cout<<n<<" "; } cout<<"\nA constant iterator of an elements are : "; for(auto i = num_list.cbegin(); i!=num_list.end(); i++) { cout<<*i<<" "; } }
输出
执行上述程序后,它将产生以下输出:
List elements are: 1 2 3 4 5 A constant iterator of an elements are : 1 2 3 4 5