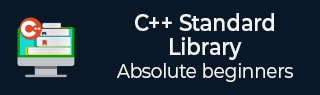
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ List::emplace() 函数
C++ 的 std::list::emplace() 函数用于在容器中 `pos` 位置(`pos` 是一个迭代器,新元素将在此之前构造)之前直接插入一个新元素。
使用内置函数 `begin()` 和 `end()`,您可以指定容器中元素的起始和结束位置,并使用 `emplace()` 函数插入元素。插入新元素后,列表大小将增加一。如果我们给出像 (0,1,2,3..n) 这样的位置值,则会抛出错误。
语法
以下是 C++ std::list::emplace() 函数的语法:
iterator emplace (const_iterator position, val);
参数
- pos − 列表中插入新元素的位置。
- val − 指定要插入到列表中的新元素值。
返回值
此函数返回一个指向新放置元素的随机访问迭代器。
示例 1
在列表的末尾(或最后一个位置)插入一个新元素。
在下面的程序中,我们使用 C++ std::list::emplace() 函数在指定位置(末尾)在当前列表 {10, 20, 30} 中插入一个新元素值 40。
#include<iostream> #include<list> using namespace std; int main() { //create a list list<int> num_list = {10, 20, 30}; cout<<"List elements before emplace operation: "; for(int n : num_list) { cout<<n<<" "; } //using emplace() function num_list.emplace(num_list.end(), 40); cout<<"\nList elements after emplace operation: "; for(int n : num_list) { cout<<n<<" "; } return 0; }
输出
这将生成以下输出:
List elements before emplace operation: 10 20 30 List elements after emplace operation: 10 20 30 40
示例 2
在列表的开头(或第一个位置)插入一个新元素。
以下是 C++ std::list::emplace() 函数的另一个示例。在这里,我们创建一个名为 symbols 的列表(类型为 char),其值为 {'@', '#', '$', '%' }。然后使用 emplace() 函数,我们尝试在此列表的指定位置(开头)插入一个新的 char 元素 '&'。
#include<iostream> #include<list> using namespace std; int main() { //create a list list<char> symbols = {'@', '#', '$', '%'}; cout<<"List elements before emplace operation: "; for(char n : symbols) { cout<<n<<" "; } //using emplace() function symbols.emplace(symbols.begin(), '&'); cout<<"\nList elements after emplace operation: "; for(char n : symbols) { cout<<n<<" "; } return 0; }
输出
以下是上述程序的输出:
List elements before emplace operation: @ # $ % List elements after emplace operation: & @ # $ %
示例 3
在空列表中插入元素。
在此示例中,我们创建一个名为 cities 的列表(类型为 string),其值为 {"Delhi", "Mumbai", "Hyderabad", "Lucknow"}。然后,使用 emplace() 函数,我们尝试在此列表的指定位置(末尾)插入字符串值 "Prayagraj"。
#include<iostream> #include<list> using namespace std; int main() { //create a list list<string> cities = {"Delhi", "Mumbai", "Hyderabad", "Lucknow"}; cout<<"List elements before emplace operation: "; for(string c : cities) { cout<<c<<" "; } //using emplace() function cities.emplace(cities.end(), "Prayagraj"); cout<<"\nList elements after emplace operation: "; for(string c : cities) { cout<<c<<" "; } return 0; }
输出
执行上述程序后,它将产生以下输出:
List elements before emplace operation: Delhi Mumbai Hyderabad Lucknow List elements after emplace operation: Delhi Mumbai Hyderabad Lucknow Prayagraj
示例 4
动态地在列表中插入元素。
在下面的程序中,我们创建一个值为 {1,2,3} 的列表(类型为 int)。然后在 for 循环内使用 emplace() 函数在指定位置(末尾)动态地将元素插入当前列表。
#include<iostream> #include<list> using namespace std; int main() { //create a list list<int> num_list = {1,2,3}; cout<<"List elements before emplace operation: "; for(int n : num_list) { cout<<n<<" "; } //using for loop for(int i = 3; i<10; i++) { num_list.emplace(num_list.end(), i+1); } cout<<"\nList elements after emplace operation: "; for(int l : num_list) { cout<<l<<" "; } return 0; }
输出
上述程序生成以下输出:
List elements before emplace operation: 1 2 3 List elements after emplace operation: 1 2 3 4 5 6 7 8 9 10