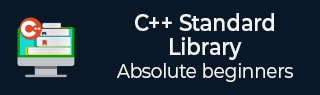
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ List::emplace_front() 函数
C++ 的 std::list::emplace_front() 函数用于在列表开头插入新元素。
它将指定的值插入到当前列表的开头,并将列表大小增加一。emplace_front() 函数的返回类型为 void,这意味着它不返回值。
emplace_front() 函数类似于 push_front() 函数,push_front() 函数插入给定元素并在列表开头添加对象的副本,而 emplace_front() 函数插入元素并在列表开头就地构造对象,通过避免复制操作,可能会提高性能。
语法
以下是 std::list::emplace_front() 函数的语法:
void emplace_front (val);
参数
- val − 要插入到列表中的新元素值。
返回值
此函数不返回值。
示例 1
在下面的程序中,我们使用 C++ std::list::emplace_front() 函数在当前列表 {2, 3, 4, 5} 的开头插入新元素值 1。
#include<iostream> #include<list> using namespace std; int main() { //create a list list<int> num_list = {2, 3, 4, 5}; cout<<"List elements before emplace_front operation: "; for(int n : num_list) { cout<<n<<" "; } //element value int val = 1; //using emplace_front() function num_list.emplace_front(val); cout<<"\nList elements after emplace_front() operation: "<<endl; for(int n : num_list) { cout<<n<<" "; } return 0; }
输出
以上程序产生以下输出:
List elements before emplace_front operation: 2 3 4 5 List elements after emplace_front() operation: 1 2 3 4 5
示例 2
除了 int 元素之外,您还可以将 char 元素插入列表(char 类型)。
以下是 C++ std::list::emplace_front() 函数的另一个示例。在这里,我们创建一个名为 vowels 的列表(char 类型),其值为 {'e', 'i', 'o', 'u'}。然后,使用 emplace_front() 函数,我们尝试在这个列表的开头插入一个新的 char 元素 'a'。
#include<iostream> #include<list> using namespace std; int main() { //create a list list<char> vowels = {'e', 'i', 'o', 'u'}; cout<<"List elements before emplace_front operation: "; for(char v : vowels) { cout<<v<<" "; } //element value char val = 'a'; //using emplace_front() function vowels.emplace_front(val); cout<<"\nList elements after emplace_front() operation: "<<endl; for(char v : vowels) { cout<<v<<" "; } return 0; }
输出
执行上述程序后,将生成以下输出:
List elements before emplace_front operation: e i o u List elements after emplace_front() operation: a e i o u
示例 3
在列表(string 类型)中插入字符串元素。
在这个例子中,我们创建一个名为 fruits 的列表(string 类型),其值为 {"Orange", "Banana", "Grapes", "Apple"}。然后,使用 emplace_front() 函数,我们尝试在这个列表的开头插入字符串元素 "Papaya"。
#include<iostream> #include<list> using namespace std; int main() { //create a list list<string> fruits = {"Orange", "Banana", "Grapes", "Apple"}; cout<<"List elements before emplace_front operation: "; for(string f : fruits) { cout<<f<<" "; } //element value string val = "Papaya"; //using emplace_front() function fruits.emplace_front(val); cout<<"\nList elements after emplace_front() operation: "<<endl; for(string f : fruits) { cout<<f<<" "; } return 0; }
输出
这将生成以下输出:
List elements before emplace_front operation: Orange Banana Grapes Apple List elements after emplace_front() operation: Papaya Orange Banana Grapes Apple
示例 4
在下面的示例中,我们创建一个名为 numbers 的列表(int 类型),其值为 {5,6,7,8,9,10}。然后,我们在 for 循环内使用 emplace_front() 函数动态地在当前列表的开头插入数字。
#include<iostream> #include<list> using namespace std; int main() { //create a list list<int> numbers = {5,6,7,8,9,10}; cout<<"List elements before emplace_front operation: "; for(int n : numbers ) { cout<<n<<" "; } //using emplace_front() function for(int i = 5; i>=1; i--) { numbers.emplace_front(i); } cout<<"\nList elements after emplace_front operation: "<<endl; for(int n : numbers ) { cout<<n<<" "; } return 0; }
输出
以下是上述程序的输出:
List elements before emplace_front operation: 5 6 7 8 9 10 List elements after emplace_front operation: 1 2 3 4 5 5 6 7 8 9 10
广告