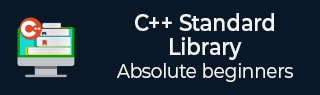
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ List::empty() 函数
C++ 的std::list::empty()函数用于检查列表是否为空。
当且仅当当前列表为空时,它返回布尔值 true;否则返回 false。在 C++ 中,bool 数据类型与其他编程语言中的布尔数据类型一样,可以取值 true(1) 或 false(0)。它在结果中返回 1 表示 true,返回 0 表示 false 条件。
语法
以下是 C++ std::list::empty() 函数的语法:
bool empty() const;
参数
- 它不接受任何参数。
返回值
如果列表为空,则此函数返回 true;否则返回 false。
示例 1
如果当前列表不为空,则 empty() 函数返回 0。
在下面的程序中,我们使用 C++ std::list::empty() 函数来验证当前列表 {1, 2,3 4, 5} 是否为空。
#include<iostream> #include<list> using namespace std; int main(void) { //create an integer list list<int> lst = {1, 2, 3, 4, 5}; cout<<"The list elements are: "; for(int l: lst){ cout<<l<<" "; } cout<<"\nIs list is empty or not? "<<lst.empty(); }
输出
以下是上述程序的输出:
The list elements are: 1 2 3 4 5 Is list is empty or not? 0
示例 2
如果当前列表为空,则 empty() 函数返回 1。
以下是 C++ std::list::empty() 函数的另一个示例,这里我们创建一个空列表(类型为 char),并使用 empty() 函数尝试验证此列表是否为空。
#include<iostream> #include<list> using namespace std; int main(void) { list<char> lst = {}; cout<<"The list elements are: "; for(char l: lst){ cout<<l<<" "; } cout<<"\nThe list size is: "<<lst.size(); cout<<"\nIs list is empty or not? "<<lst.empty(); }
输出
上述程序产生以下输出:
The list elements are: The list size is: 0 Is list is empty or not? 1
示例 3
使用条件语句检查列表是否为空。
在下面的示例中,我们使用 empty() 函数的结果在条件语句中检查名为 cities {"Delhi", "Hyderabad", "Lucknow", "Prayagraj"} 的当前列表是否为空。
#include<iostream> #include<list> using namespace std; int main(void) { list<string> cities = {"Delhi", "Hyderabad", "Lucknow", "Prayagraj"}; cout<<"The list elements are: "; for(string l: cities){ cout<<l<<" "; } cout<<"\nThe list size is: "<<cities.size(); int res = cities.empty(); if(res==1){ cout<<"\nList is an empty list."; } else{ cout<<"\nList is not empty."; } }
输出
执行上述程序后,它会产生以下输出:
The list elements are: Delhi Hyderabad Lucknow Prayagraj The list size is: 4 List is not empty.
示例 4
在此示例中,我们创建一个名为 marks 的列表,其值为 {80,90,70,95,88,78}。使用 clear() 函数,我们通过删除所有元素来清除列表,然后我们在条件语句中使用 empty() 函数的结果来验证列表是否为空。
#include<iostream> #include<list> using namespace std; int main(void) { list<int> marks = {80,90,70,95,88,78}; cout<<"The list elements are: "; for(int l: marks){ cout<<l<<" "; } cout<<"\nThe list size is: "<<marks.size(); //clear the list marks.clear(); cout<<"\nAfter clear the list size is: "<<marks.size(); int res = marks.empty(); if(res==1){ cout<<"\nList is an empty list."; } else{ cout<<"\nList is not empty."; } }
输出
执行上述程序后,它会生成以下输出:
The list elements are: 80 90 70 95 88 78 The list size is: 6 After clear the list size is: 0 List is an empty list.
广告