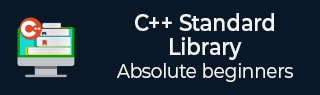
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ List::get_allocator() 函数
C++ 的std::list::get_allocator()函数用于检索与列表关联的分配器。
在 C++ 中,分配器用于标准库来处理存储在容器中的元素的分配和释放。分配是请求访问数据集的过程。如果您分配一个存在的数据集,系统允许您打开数据集;如果您分配一个不存在的数据集,系统会在可用设备上为其创建空间,并允许您打开该空间。
语法
以下是 C++ std::list::get_allocator() 的语法:
allocator_type get_allocator() const;
参数
- 它不接受任何参数。
返回值
此函数返回与列表关联的分配器。
示例 1
在下面的程序中,我们使用 C++ std::list::get_allocator() 函数来检索与当前列表 {10, 20, 30, 40, 50} 关联的分配器。
#include<iostream> #include<list> using namespace std; int main(void) { //create a list list<int> num_list = {10, 20, 30, 40, 50}; cout<<"List elements are: "; for(int i : num_list){ cout<<i<<" "; } int *p = NULL; p = num_list.get_allocator().allocate(5); for (int i = 0; i<5; ++i) p[i] = i + 1; cout<<"\nList contains following elements"<<endl; for (int i = 0; i<5; ++i) cout<<p[i]<<" "; return 0; }
输出
以下是上述程序的输出:
List elements are: 10 20 30 40 50 List contains following elements 1 2 3 4 5
示例 2
以下是 C++ std::list::get_allocator() 函数的另一个示例。在这里,我们创建一个名为 num_list 的列表(int 类型)。然后,使用 get_allocator() 函数,我们尝试检索与当前列表关联的分配器。
#include<iostream> #include<list> using namespace std; int main(void) { //create a list list<int> num_list; //creating array using this list get_allocator int *arr; arr = num_list.get_allocator().allocate(5); arr[0] = 10; arr[1] = 20; arr[2] = 30; arr[3] = 40; arr[4] = 50; cout<<"List contains the following elements: "; for(int i = 0; i<5; i++) { cout<<arr[i]<<" "; } }
输出
这将生成以下输出:
List contains the following elements: 10 20 30 40 50
示例 3
在此示例中,我们创建一个名为 char_list 的列表(char 类型),其值为 空。然后,使用 get_allocator() 函数,我们尝试获取与该列表关联的分配器。我们使用 allocate() 函数指定要分配的对象数量为 5,以便为指向附近内存位置的指针分配存储空间。
#include<iostream> #include<list> using namespace std; int main(void) { //create a list list<char> char_list = {}; cout<<"Size of list: "<<char_list.size()<<endl; //creating array using this list get_allocator char *char_arr; char_arr = char_list.get_allocator().allocate(3); char_arr[0] = 'a'; char_arr[1] = 'b'; char_arr[2] = 'c'; cout<<"List contains the following elements: "; for(int i = 0; i<5; i++) { cout<<char_arr[i]<<" "; } }
输出
执行上述程序后,将产生以下输出:
Size of list: 0 List contains the following elements: a b c ..
广告