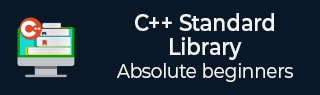
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ List::max_size() 函数
C++ 的std::list::max_size()函数用于获取列表的最大大小。
它返回当前列表可以容纳的最大元素数(或最大大小)。换句话说,它检索容器可以达到的最大大小,但是,不能保证它可以分配该大小的元素,并且仍然可能无法为列表容器的特定点分配存储空间。此值取决于系统或库的实现。
语法
以下是 C++ std::list::max_size() 函数的语法:
size_type max_size();
参数
- 它不接受任何参数。
返回值
此函数返回可以放入列表中的最大数字。
示例 1
如果列表是 int 类型。
在下面的程序中,我们使用 C++ std::list::max_size() 函数获取名为 num_list 的当前列表的最大大小。
#include<iostream> #include<list> using namespace std; int main() { //create a list list<int> num_list; cout<<"Size of the list: "<<num_list.size()<<endl; cout<<"The max_size of list = "<<num_list.max_size()<<endl; return 0; }
输出
执行上述程序后,将产生以下输出:Size of the list: 0 The max_size of list = 384307168202282325
示例 2
查找 char 类型列表的最大大小。
以下是 C++ std::list::max_size() 函数的另一个示例。在这里,我们创建了一个名为 char_list 的列表(类型为 char),其值为 {'a', 'b', 'c', 'd'}。然后,使用 max_size() 函数,我们尝试查找当前列表的最大大小。
#include<iostream> #include<list> using namespace std; int main() { //create a list list<char> char_list = {'a', 'b', 'c', 'd'}; cout<<"Size of the list: "<<char_list.size()<<endl; cout<<"List elements are: "<<endl; for(char c: char_list) { cout<<c<<" "; } cout<<"\nThe max_size of list = "<<char_list.max_size()<<endl; return 0; }
输出
以下是上述程序的输出:
Size of the list: 4 List elements are: a b c d The max_size of list = 384307168202282325
示例 3
除了 int 类型和 char 类型列表之外,您还可以找到 string 类型列表的最大大小。
在此示例中,我们创建了一个名为 str_list 的列表(类型为 string),其值为 {"Java", "C++", "Python", "Apex"}。然后,使用 max_size() 函数,我们尝试查找此列表的最大大小。
#include<iostream> #include<list> using namespace std; int main() { //create a list list<string> str_list = {"Java", "C++", "Python", "Apex"}; cout<<"Size of the list: "<<str_list.size()<<endl; cout<<"List elements are: "<<endl; for(string s: str_list) { cout<<s<<" "; } cout<<"\nThe max_size of list = "<<str_list.max_size()<<endl; return 0; }
输出
这将生成以下输出:
Size of the list: 4 List elements are: Java C++ Python Apex The max_size of list = 192153584101141162
广告