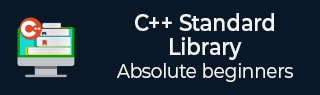
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ List::pop_front() 函数
C++ 的std::list::pop_front()函数用于移除列表的第一个元素。它不接受任何参数,移除(或弹出)当前列表的第一个元素,并将列表大小减少一个。pop_front() 函数不返回值,因为函数返回类型为 void。
语法
以下是 C++ std::list::void pop_front() 函数的语法:
void pop_front();
参数
- 它不接受任何参数。
返回值
此函数不返回值。
示例 1
在以下程序中,我们使用 C++ std::list::pop_front() 函数来移除(或弹出)当前列表 {1, 2, 3, 4, 5, 6} 的第一个元素 1。
#include<iostream> #include<list> using namespace std; int main() { list<int> lst = {1, 2, 3, 4, 5, 6}; cout<<"The list elements before the pop_front() operation: "<<endl; for(int l : lst) { cout<<l<<" "; } lst.pop_front(); cout<<"\nThe list elements after the pop_front() operation: "; for(int l1 : lst) { cout<<l1<<" "; } }
输出
以下是上述程序的输出:
The list elements before the pop_front() operation: 1 2 3 4 5 6 The list elements after the pop_front() operation: 2 3 4 5 6
示例 2
除了整数元素外,您还可以从列表中移除第一个字符元素(类型为 char)。
以下是 C++ std::list::pop_front() 函数的另一个示例。在这里,我们创建一个包含元素 {'A', 'B', 'C', 'D', 'E'} 的列表(类型为 char)。然后,使用 pop_front() 函数,我们尝试从该列表中移除第一个元素 'A'。
#include<iostream> #include<list> using namespace std; int main() { list<char> lst = {'A', 'B', 'C', 'D', 'E'}; cout<<"The list elements before the pop_front() operation: "<<endl; for(char l : lst) { cout<<l<<" "; } lst.pop_front(); cout<<"\nThe list elements after the pop_front() operation: "; for(char l1 : lst) { cout<<l1<<" "; } }
输出
执行上述程序后,将产生以下输出:
The list elements before the pop_front() operation: A B C D E The list elements after the pop_front() operation: B C D E
示例 3
您还可以从列表(类型为 string)中移除第一个字符串。
在此示例中,我们创建一个名为 names 的列表(类型为 string),其值为 {"Rohan", "Geeta", "Rahul", "Sonu", "Vinod"}。然后,使用 std::list::pop_front() 函数,我们尝试从该列表中移除第一个元素 "Rohan"。
#include<iostream> #include<list> using namespace std; int main() { list<string> names = {"Rohan", "Geeta", "Rahul", "Sonu", "Vinod"}; cout<<"The list elements before the pop_front() operation: "<<endl; for(string l : names) { cout<<l<<" "; } names.pop_front(); cout<<"\nThe list elements after the pop_front() operation: "; for(string l1 : names) { cout<<l1<<" "; } }
输出
上述程序生成以下输出:
The list elements before the pop_front() operation: Rohan Geeta Rahul Sonu Vinod The list elements after the pop_front() operation: Geeta Rahul Sonu Vinod
示例 4
如果列表为空且包含空格,则 pop_front() 函数会从列表中移除空格,并将列表的大小减少一个。
#include<iostream> #include<list> using namespace std; int main() { list<string> colors = {" "}; cout<<"The list elements before the pop_front() operation: "<<endl; cout<<"List size before the pop_front() operation: "<<colors.size()<<endl; for(string l : colors) { cout<<l<<" "; } colors.pop_front(); cout<<"\nThe list elements after the pop_front() operation: "; for(string l1 : colors) { cout<<l1<<" "; } cout<<"\nList size after the pop_front() operation: "<<colors.size(); }
输出
执行上述程序后,将产生以下输出:
The list elements before the pop_front() operation: List size before the pop_front() operation: 1 The list elements after the pop_front() operation: List size after the pop_front() operation: 0
广告