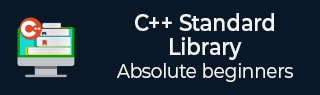
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ List::remove() 函数
C++ 的 std::list::remove() 函数用于从列表中删除元素。它接受一个参数作为任意类型(int、char、string 等),并从当前列表中移除与指定元素匹配的元素;否则,当前列表不会发生任何变化。它会根据从列表中移除的元素数量减少列表的大小。它不返回任何值,因为函数的返回类型是 void。
语法
以下是 C++ std::list::remove() 函数的语法:
void remove (const value_type& val);
参数
- val − 要移除的元素的值。
返回值
此函数不返回任何值。
示例 1
如果给定元素存在于列表中。
在下面的程序中,我们使用 C++ std::list::remove() 函数从当前列表 {10, 20, 30, 40, 50, 60} 中移除指定的元素 20(如果匹配)。
#include<iostream> #include<list> using namespace std; int main(void) { list<int> lst = {10, 20, 30, 40, 50, 60}; cout<<"The list elements before the remove operation: "<<endl; for(int l : lst){ cout<<l<<" "; } int ele = 10; cout<<"\nThe element value: "<<ele; //using the remove() function lst.remove(10); cout<<"\nThe list elements after the remove operation: "; for(int l1 : lst){ cout<<l1<<" "; } }
输出
执行上述程序后,将产生以下输出:
The list elements before the remove operation: 10 20 30 40 50 60 The element value: 10 The list elements after the remove operation: 20 30 40 50 60
示例 2
如果给定元素不存在于列表中,remove() 函数不会从列表中移除任何元素,当前列表不会发生任何变化。
以下是 std::list::remove() 函数的另一个示例,在这里,我们创建一个包含值 {'A', 'B', 'C', 'D'} 的列表(类型为 char),并使用此函数尝试移除指定元素 'E'(如果匹配)。
#include<iostream> #include<list> using namespace std; int main(void) { list<char> lst = {'A', 'B', 'C', 'D'}; cout<<"The list elements before the remove operation: "<<endl; for(char l : lst){ cout<<l<<" "; } char ele = 'E'; cout<<"\nThe element value: "<<ele; //using the remove() function lst.remove(ele); cout<<"\nThe list elements after the remove operation: "; for(char l1 : lst){ cout<<l1<<" "; } }
输出
以下是上述程序的输出:
The list elements before the remove operation: A B C D The element value: E The list elements after the remove operation: A B C D
示例 3
您还可以从列表(类型为 string)中移除字符串值。
在此示例中,我们创建一个名为 fruits 的列表(类型为 string),其值为 {"Mango", "Banana", "Apple", "Grapes"}。然后,使用 std::list::remove() 函数,我们尝试移除此列表中的元素 'Apple'(如果存在)。
#include<iostream> #include<list> using namespace std; int main(void) { list<string> fruits = {"Mango", "Banana", "Apple", "Grapes"}; cout<<"The list elements before the remove operation: "<<endl; for(string l : fruits){ cout<<l<<" "; } string ele = "Apple"; cout<<"\nThe element value: "<<ele; //using the remove() function fruits.remove(ele); cout<<"\nThe list elements after the remove operation: "; for(string l1 : fruits){ cout<<l1<<" "; } }
输出
上述程序生成以下输出:
The list elements before the remove operation: Mango Banana Apple Grapes The element value: Apple The list elements after the remove operation: Mango Banana Grapes
广告