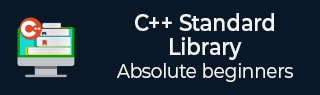
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ List::remove_if() 函数
C++ 的std::list::remove_if()函数用于移除列表中满足特定条件的元素。它接受一个名为 `pred` 的参数,并移除列表中所有满足该条件的元素。`pred` 是一个谓词函数,它是用户定义的函数,只接受一个参数,该参数的类型必须与列表中存储的项的类型匹配。
remove_if() 函数不返回任何值,因为函数的返回类型是 void,它会移除列表中所有谓词函数返回 true 的元素。
语法
以下是 C++ std::list::remove_if() 函数的语法:
void remove_if (Predicate pred);
参数
pred − 这是一个一元谓词函数,它接受相同类型的数值并返回 true,表示这些值将从列表中移除。
返回值
此函数不返回任何值。
示例 1
在下面的程序中,我们定义了一个名为 Con(int n) 的谓词函数,它接受一个 int 类型参数,我们使用此函数过滤掉所有大于 2 的值。然后,我们将此函数作为参数传递给 remove_if() 函数,以移除列表中所有大于 2 的元素。
#include<iostream> #include<list> using namespace std; //custom function to check condition bool Con(int n) { return (n>2); } int main(void) { list<int> num_list = {1, 2, 3, 4, 5}; cout<<"List elements before remove_if operation: "<<endl; for(int n : num_list) { cout<<n<<" "; } //using remove_if() function num_list.remove_if(Con); cout<<"\nList elements after remove_if operation: "; for(int n : num_list) { cout<<n<<" "; } return 0; }
输出
这将生成以下输出:
List elements before remove_if operation: 1 2 3 4 5 List elements after remove_if operation: 1 2
示例 2
以下是 C++ std::list::remove_if() 函数的另一个示例。在这里,我们定义了一个名为 filter_length(string len) 的谓词函数,它接受一个字符串作为参数。然后,我们将此函数作为参数传递给 remove_if() 函数,以移除列表中长度大于 3 的所有字符串元素。
#include<iostream> #include<list> using namespace std; bool filter_length(string n) { return (n.length()>3); } int main(void) { list<string> num_list = {"Hello", "how", "are", "you"}; cout<<"List elements before remove_if operation: "<<endl; for(string n : num_list) { cout<<n<<" "; } //using remove_if() function num_list.remove_if(filter_length); cout<<"\nList elements after remove_if operation: "; for(string n : num_list) { cout<<n<<" "; } return 0; }
输出
以下是上述程序的输出:
List elements before remove_if operation: Hello how are you List elements after remove_if operation: how are you
示例 3
从列表中移除偶数。
在此示例中,我们定义了一个名为 filter_evens(int n) 的谓词函数,它接受一个 int 类型参数。然后,我们将此函数作为参数传递给 remove_if() 函数,以从当前列表 {1,2,3,4,5,6,7,8,9,10} 中移除所有偶数元素(或数字)。
#include<iostream> #include<list> using namespace std; //custom function to check condition bool filter_evens(int n) { return (n%2 == 0); } int main(void) { list<int> num_list = {1,2,3,4,5,6,7,8,9,10}; cout<<"List elements before remove_if operation: "<<endl; for(int n : num_list) { cout<<n<<" "; } //using remove_if() function num_list.remove_if(filter_evens); cout<<"\nAfter removing even numbers from list elements are: "; for(int n : num_list) { cout<<n<<" "; } return 0; }
输出
执行上述程序后,它将产生以下输出:
List elements before remove_if operation: 1 2 3 4 5 6 7 8 9 10 After removing even numbers from list elements are: 1 3 5 7 9
广告