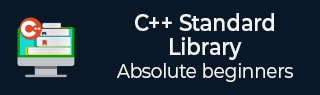
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ List::reverse() 函数
C++ 的 std::list::reverse() 函数用于反转列表中元素的顺序。
它不接受任何参数,并反转列表容器中元素的顺序。例如,假设我们有一个值为 {1,2,3,4} 的列表,如果我们尝试反转列表元素的顺序,则最后一个元素将放置在第一个位置,倒数第二个元素将放置在第二个位置,依此类推。最终,输出将显示为 {4,3,2,1}。此函数的返回类型为 void,这意味着它不返回值。
语法
以下是 C++ std::list::void reverse() 函数的语法:
void reverse();
参数
- 它不接受任何参数。
返回值
此函数不返回值。
示例 1
在下面的程序中,我们使用 C++ std::list::reverse() 函数来反转当前列表 {10,20, 30, 40} 中元素的顺序。
#include<iostream> #include<list> using namespace std; int main() { list<int> num_list = {10,20, 30, 40}; cout<<"The list elements before the reverse() operation: "<<endl; for(int lst : num_list) { cout<<lst<<" "; } //use the reverse() function num_list.reverse(); cout<<"\nThe list elements after the reverse() operation: "; for(int lst1 : num_list) { cout<<lst1<<" "; } }
输出
上述程序产生以下输出:
The list elements before the reverse() operation: 10 20 30 40 The list elements after the reverse() operation: 40 30 20 10
示例 2
除了 int 元素外,您还可以反转列表中 char 元素(char 类型)的顺序。
以下是 C++ std::list::reverse() 函数的另一个示例。在这里,我们创建一个包含元素 {'O', 'L', 'L', 'H', 'E'} 的列表(char 类型)。然后,使用 reverse() 函数,我们尝试反转当前列表中元素的顺序。
#include<iostream> #include<list> using namespace std; int main() { list<char> char_list = {'O', 'L', 'L', 'E', 'H'}; cout<<"The list elements before the reverse() operation: "<<endl; for(char lst : char_list) { cout<<lst<<" "; } //using the reverse() function char_list.reverse(); cout<<"\nThe list elements after the reverse() operation: "; for(char lst1 : char_list) { cout<<lst1<<" "; } }
输出
执行上述程序后,它将产生以下输出:
The list elements before the reverse() operation: O L L E H The list elements after the reverse() operation: H E L L O
示例 3
您还可以反转列表中字符串元素(string 类型)的顺序。
在这个程序中,我们创建了一个包含元素 {"Welcome", "to", "Tutorials", "Point"} 的列表(string 类型)。然后,使用 reverse() 函数,我们尝试反转此列表中元素的顺序。
#include<iostream> #include<list> using namespace std; int main() { list<string> msg = {"Welcome", "to", "Tutorials", "Point"}; cout<<"The list Elements before the reverse() operation: "<<endl; for(string l1: msg) { cout<<l1<<" "; } //using the reverse() function msg.reverse(); cout<<"\nThe list elements after the reverse() function: "; for(string l2: msg){ cout<<l2<<" "; } return 0; }
输出
这将生成以下输出:
The list Elements before the reverse() operation: Welcome to Tutorials Point The list elements after the reverse() function: Point Tutorials to Welcome
广告