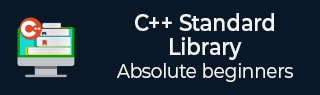
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ List::swap() 函数
C++ 的std::list::swap()函数用于交换列表的内容与其他列表的内容。它不会对单个列表元素调用任何移动、复制或交换操作。
在 C++ 中,交换只不过是一种交换功能,用于将数据与另一个数据交换。swap() 函数在交换列表元素时会忽略列表大小,但会验证列表类型。如果列表类型不同,它将引发错误。此函数的返回类型为 void,这意味着它不返回任何值。它根据需要更改列表大小。
语法
以下是 C++ std::list::swap() 函数的语法:
void swap( list& other );
参数
other − 它是另一个相同类型的列表对象。
返回值
此函数不返回任何值。
示例 1
在下面的程序中,我们使用 C++ std::list::swap() 函数将指定的列表(int 类型)元素 {1, 2, 3, 4, 5} 与当前列表元素 {10, 20, 30, 40} 交换。
#include<iostream> #include<list> using namespace std; int main() { //create an int list list<int> list1 = {10, 20, 30, 40}; cout<<"First list elements before swap: "<<endl; for(int l1 : list1) { cout<<l1<<" "; } list<int> list2 = {1, 2, 3, 4, 5}; cout<<"\nSecond list elements before swap: "<<endl; for(int l2: list2) { cout<<l2<<" "; } //using the swap() function list1.swap(list2); cout<<"\nFirst list elements after swap: "<<endl; for(int l1 : list1) { cout<<l1<<" "; } cout<<"\nSecond list elements after swap: "<<endl; for(int l2 : list2) { cout<<l2<<" "; } }
输出
以下是上述程序的输出:
First list elements before swap: 10 20 30 40 Second list elements before swap: 1 2 3 4 5 First list elements after swap: 1 2 3 4 5 Second list elements after swap: 10 20 30 40
示例 2
以下是 C++ std::list::swap() 函数的另一个示例。在这里,我们创建了两个名为 upper_case_list 和 lower_case_list 的列表(char 类型),其值为 {'A', 'B', 'C', 'D'} 和 {'a', 'b', 'c', 'd'}。然后,使用 swap() 函数,我们尝试将 upper_case_list 元素与 lower_case_list 元素交换。
#include<iostream> #include<list> using namespace std; int main() { //create list list<char> upper_case_list = {'A', 'B', 'C', 'D'}; cout<<"First list elements before swap: "<<endl; for(char u : upper_case_list) { cout<<u<<" "; } list<char> lower_case_list = {'a', 'b', 'c', 'd'}; cout<<"\nSecond list elements before swap: "<<endl; for(char l: lower_case_list) { cout<<l<<" "; } //using the swap() function upper_case_list.swap(lower_case_list); cout<<"\nFirst list elements after swap: "<<endl; for(char u : upper_case_list) { cout<<u<<" "; } cout<<"\nSecond list elements after swap: "<<endl; for(char l : lower_case_list) { cout<<l<<" "; } }
输出
执行上述程序后,将产生以下输出:
First list elements before swap: A B C D Second list elements before swap: a b c d First list elements after swap: a b c d Second list elements after swap: A B C D
示例 3
除了 int 和 char 列表元素外,您还可以交换列表中的字符串元素(string 类型)。
在此示例中,我们创建了两个名为 names 和 surnames 的列表(string 类型),其值为 {"Rahul", "Geeta", "Reeta"} 和 {"Verma", "Gupta", "Sharma"}。然后,使用 swap() 函数,我们尝试将 name 列表元素与 surnames 列表元素交换。
#include<iostream> #include<list> using namespace std; int main() { //create list list<string> names = {"Rahul", "Geeta", "Reeta"}; cout<<"First list elements before swap: "<<endl; for(string n : names){ cout<<n<<" "; } list<string> surnames = {"Verma", "Gupta", "Sharma"}; cout<<"\nSecond list elements before swap: "<<endl; for(string s: surnames){ cout<<s<<" "; } //using the swap() function names.swap(surnames); cout<<"\nFirst list elements after swap: "<<endl; for(string n : names){ cout<<n<<" "; } cout<<"\nSecond list elements after swap: "<<endl; for(string s : surnames){ cout<<s<<" "; } }
输出
这将生成以下输出:
First list elements before swap: Rahul Geeta Reeta Second list elements before swap: Verma Gupta Sharma First list elements after swap: Verma Gupta Sharma Second list elements after swap: Rahul Geeta Reeta