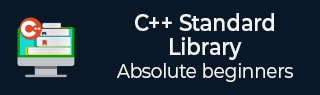
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ multimap::cbegin() 函数
C++ 的 std::multimap::cbegin() 函数用于返回指向 multimap 中第一个元素的常量迭代器,从而可以只读访问其元素。与返回可变迭代器的 begin() 函数不同,cbegin() 函数确保数据无法修改,从而提高了对其元素进行安全迭代的效率。该函数的时间复杂度为常数,即 O(1)。
语法
以下是 std::multimap::cbegin() 函数的语法。
const_iterator cbegin() const noexcept;
参数
此函数不接受任何参数。
返回值
此函数返回一个指向第一个元素的常量迭代器。
示例
让我们看下面的例子,我们将使用 cbegin() 函数并访问第一个元素。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{1, "Welcome"}, {2, "Hi"}, {3, "Hello"}}; auto it = a.cbegin(); std::cout << " " << it->first << ": " << it->second << std::endl; return 0; }
输出
以上代码的输出如下:
1: Welcome
示例
考虑以下示例,我们将使用 cbegin() 函数查找特定元素。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{1, "Hi"}, {2, "Hello"}, {3, "Namaste"}}; auto x = a.cbegin(); while (x != a.cend() && x->first != 3) { ++x; } if (x != a.cend()) { std::cout << "Element found: " << x->second << std::endl; } else { std::cout << "Element not found." << std::endl; } return 0; }
输出
以下是以上代码的输出:
Element found: Namaste
示例
在以下示例中,我们将使用 cbegin() 函数遍历 multimap。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{1, "TutorialsPoint"}, {2, "TP"}, {3, "Tutorix"}}; for(auto x = a.cbegin(); x != a.cend(); ++x) { std::cout << x->first << ": " << x->second << std::endl; } return 0; }
输出
如果我们运行以上代码,它将生成以下输出:
1: TutorialsPoint 2: TP 3: Tutorix
multimap.htm
广告