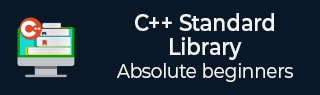
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ multimap::end() 函数
C++ 的 std::multimap::end() 函数用于返回一个指向容器末尾元素之后位置的迭代器。此元素充当占位符,不能用于解引用。它主要用于在迭代期间指示 multimap 的结尾,允许函数正确地循环遍历所有元素。返回的迭代器用于确定迭代或元素搜索何时停止,确保已处理所有元素范围。
语法
以下是 std::multimap::end() 函数的语法。
iterator end() noexcept; const_iterator end() const noexcept;
参数
它不接受任何参数。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
返回值
此函数返回一个指向末尾元素之后位置的迭代器。
示例
让我们看下面的示例,我们将演示 end() 函数的基本用法。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; a.insert({1, "A"}); a.insert({2, "B"}); a.insert({3, "C"}); std::multimap<int, std::string>::iterator x = a.end(); for (x = --a.end(); x != --a.begin(); --x) { std::cout << x->first << " => " << x->second << std::endl; } return 0; }
输出
以上代码的输出如下:
3 => C 2 => B 1 => A
示例
考虑下面的示例,我们将检查 multimap 是否为空。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; if (a.begin() == a.end()) { std::cout << "Multimap is empty." << std::endl; } else { std::cout << "Multimap is not empty." << std::endl; } return 0; }
输出
以上代码的输出如下:
Multimap is empty.
示例
在下面的示例中,我们将删除具有特定键的元素,并使用 end() 函数迭代并打印剩余的元素。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{3, "Cruze"}, {2, "Beats"}, {1, "Sail"}}; a.erase(1); for (auto x = a.begin(); x != a.end(); ++x) { std::cout << x->first << " => " << x->second << std::endl; } return 0; }
输出
让我们编译并运行以上程序,这将产生以下结果:
2 => Beats 3 => Cruze
示例
下面的示例将使用 find() 定位键,如果找到键则打印元素,否则检查 end() 以确认元素不存在。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{1, "A"}, {2, "B"}, {3, "C"}}; auto x = a.find(3); if (x != a.end()) { std::cout << "Element Found : " << x->first << " => " << x->second << std::endl; } else { std::cout << "Element Not Found :" << std::endl; } return 0; }
输出
如果我们运行以上代码,它将生成以下输出:
Element Not Found
multimap.htm
广告