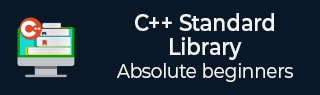
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ multimap::find() 函数
C++ 的std::multimap::find() 函数用于在 multimap 中搜索特定键。它返回一个指向键首次出现的迭代器,如果未找到则返回 end()。与 map 不同,multimap 允许重复键。它通常用于检索与特定键关联的元素,从而可以轻松访问映射到该键的所有值。此函数的时间复杂度是对数的,即 O(log n)。
语法
以下是 std::multimap::find() 函数的语法。
iterator find (const key_type& k); const_iterator find (const key_type& k) const;
参数
- k - 表示要搜索的键。
返回值
如果找到具有特定键的元素,则此函数返回指向该元素的迭代器。
示例
让我们看下面的例子,我们将演示 find() 函数的使用。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{1, "A"}, {2, "B"}, {3, "C"}}; auto x = a.find(1); if (x != a.end()) { std::cout << "Key Found : " << x->second << std::endl; } else { std::cout << "Key Not Found." << std::endl; } return 0; }
输出
以上代码的输出如下:
Key Found : A
示例
考虑另一种情况,我们将检索与键关联的多个值。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{3, "Hi"}, {3, "Hello"}, {2, "Welcome"}, {3, "Vanakam"}}; auto x = a.equal_range(3); for (auto y = x.first; y != x.second; ++y) { std::cout << "" << y->second << std::endl; } return 0; }
输出
以下是以上代码的输出:
Hi Hello Vanakam
示例
在以下示例中,我们将找到键并修改关联的值。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{1, "A"}, {2, "B"}, {3, "C"}}; auto x = a.find(1); if (x != a.end()) { x->second = "D"; std::cout << "After Modification Value Is: " << x->second << std::endl; } else { std::cout << "Key Not Found." << std::endl; } return 0; }
输出
如果我们运行以上代码,它将生成以下输出:
After Modification Value Is: D
multimap.htm
广告