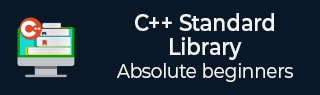
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ multimap::operator=() 函数
C++ 的std::multimap::operator=()函数用于将一个 multimap 的内容赋值给另一个 multimap,从而实现高效地复制键值对。当调用此函数时,它会将源 multimap 中的所有元素复制到目标 multimap,并保持排序顺序。
此函数有 3 个多态变体:使用复制版本、移动版本和初始化列表版本(您可以在下面找到所有变体的语法)。
此函数不会合并元素,它会用源 multimap 的内容替换目标 multimap 的内容。
语法
以下是 std::multimap::operator=() 函数的语法。
multimap& operator= (const multimap& x); or multimap& operator= (multimap&& x); or multimap& operator= (initializer_list<value_type> il);
参数
- x - 指示另一个相同类型的 multimap 对象。
- il - 指示一个 initializer_list 对象。
返回值
此函数返回 this 指针。
示例
让我们看下面的例子,我们将演示 operator=() 函数的使用。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; a.insert({1, "TP"}); a.insert({2, "TutorialsPoint"}); std::multimap<int, std::string> b; b = a; for (const auto& pair : b) { std::cout << pair.first << ": " << pair.second << std::endl; } return 0; }
输出
以上代码的输出如下:
1: TP 2: TutorialsPoint
示例
考虑下面的例子,我们将进行自赋值并观察输出。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{1, "Hi"}, {1, "Hello"}}; a = a; for (const auto& pair : a) { std::cout << pair.first << " : " << pair.second << std::endl; } return 0; }
输出
以下是以上代码的输出:
1 : Hi 1 : Hello
示例
在下面的例子中,我们将应用 operator=() 将内容赋值给空的 multimap。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{3, "Cruze"}, {2, "Sail"}}; std::multimap<int, std::string> b; b = a; for (const auto& pair : b) { std::cout << pair.first << " : " << pair.second << std::endl; } return 0; }
输出
如果我们运行以上代码,它将生成以下输出:
2 : Sail 3 : Cruze
multimap.htm
广告