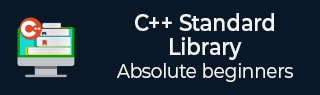
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ multimap::rbegin() 函数
C++ 的 std::multimap::rbegin() 函数用于返回一个指向最后一个元素的反向迭代器,从而可以从最后一个元素反向遍历到第一个元素。此函数对于需要反向访问元素的操作非常有用。反向迭代器可以递增以向后移动容器。此函数的时间复杂度为常数,即 O(1)。
语法
以下是 std::multimap::rbegin() 函数的语法。
reverse_iterator rbegin() nothrow; const_reverse_iterator rbegin() const nothrow;
参数
它不接受任何参数。
返回值
此函数返回一个指向容器开头的反向迭代器。
示例
让我们来看下面的例子,我们将演示 rbegin() 函数的使用。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{1, "Hi"}, {1, "Hello"}, {2, "Vanakam"}}; auto x = a.rbegin(); std::cout << x->first << ": " << x->second << std::endl; return 0; }
输出
以上代码的输出如下:
2: Vanakam
示例
考虑以下示例,我们将使用反向迭代器搜索和打印特定值。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; a.insert({1, "AB"}); a.insert({2, "BC"}); auto x = a.rbegin(); while (x != a.rend()) { if (x->second == "AB") { std::cout << "Founded Key Value Is : " << x->first << " " << x->second << std::endl; break; } ++x; } return 0; }
输出
以上代码的输出如下:
Founded Key Value Is : 1 AB
示例
在下面的示例中,我们将使用反向迭代器计算 multimap 中的元素个数。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; a.insert({1, "TP"}); a.insert({2, "Tutorix"}); a.insert({3, "Tutorialspoint"}); int count = 0; for (auto x = a.rbegin(); x != a.rend(); ++x) { count++; } std::cout << "Total Elements: " << count << std::endl; return 0; }
输出
如果我们运行以上代码,它将生成以下输出:
Total Elements: 3
multimap.htm
广告