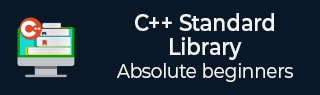
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ multimap::size() 函数
C++ 的std::multimap::size()函数用于返回multimap中元素的数量。multimap是一个关联容器,它以键值对的形式存储元素,允许具有相同键的多个元素。此函数提供了一种快速确定这些元素总数的方法。此函数的时间复杂度为常数,即 O(1)。
语法
以下是 std::multimap::size() 函数的语法。
size_type size() const noexcept;
参数
它不接受任何参数。
返回值
此函数返回容器中元素的数量。
示例
让我们来看下面的例子,我们将演示 size() 函数的使用。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; a.insert({1, "apple"}); a.insert({2, "banana"}); std::cout << "Size of the multimap is: " << a.size() << std::endl; return 0; }
输出
以下是上述代码的输出:
Size of the multimap is: 2
示例
考虑另一种情况,我们获取初始大小,然后插入一些元素并获取 multimap 的大小。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; a.insert({1, "TP"}); std::cout << "Initial size: " << a.size() << std::endl; a.insert({2, "Tutorix"}); a.insert({3, "TutorialsPoint"}); std::cout << "Size after insertion: " << a.size() << std::endl; return 0; }
输出
上述代码的输出如下:
Initial size: 1 Size after insertion: 3
示例
在下面,我们将使用 size() 函数获取 multimap 的大小,然后应用 equal_range() 函数查找具有该键的所有元素,迭代器之间的距离给出具有该键的元素的数量。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; a.insert({1, "Hi"}); a.insert({2, "Hello"}); a.insert({2, "Vanakam"}); std::cout << "Size of the multimap: " << a.size() << std::endl; auto x = a.equal_range(2); int count = std::distance(x.first, x.second); std::cout << "Elements with given key : " << count << std::endl; return 0; }
输出
如果我们运行上述代码,它将生成以下输出:
Size of the multimap: 3 Elements with given key : 2
示例
下面的示例中,我们将用 3 个元素初始化 multimap,键 1 出现两次,然后删除键 1 的元素并观察输出。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; a.insert({1, "AB"}); a.insert({1, "BC"}); a.insert({2, "CD"}); std::cout << "Size before erasing: " << a.size() << std::endl; a.erase(1); std::cout << "Size after erasing given key: " << a.size() << std::endl; return 0; }
输出
让我们编译并运行上述程序,这将产生以下结果:
Size before erasing: 3 Size after erasing given key: 1
multimap.htm
广告