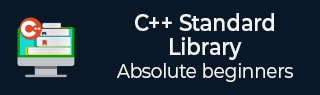
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ multimap::swap() 函数
C++ 的 std::multimap::swap() 函数用于交换两个 multimap 的内容。它交换两个 multimap 的元素、大小和分配器,而无需重新分配内存或更改单个元素。
swap() 函数可以通过两种方式调用:作为成员函数或作为非成员函数。当用作成员函数时,swap() 的时间复杂度为常数,即 O(1),否则当用作非成员函数时为线性,即 O(n)。您可以在下面找到两种方法的语法。
语法
以下是 std::multimap::swap() 函数的语法。
void swap (multimap& x); or void swap (multimap<Key,T,Compare,Alloc>& first, multimap<Key,T,Compare,Alloc>& second);
参数
- x − 表示相同类型的 multimap 对象。
- first − 表示第一个 multimap 对象
- second − 表示相同类型的第二个 multimap 对象。
返回值
此函数不返回任何值。
示例
让我们来看下面的例子,我们将演示 swap() 函数的使用。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; std::multimap<int, std::string> b; a.insert({1, "Vanakam"}); a.insert({2, "Namaste"}); b.insert({3, "Hi"}); b.insert({4, "Hello"}); a.swap(b); std::cout << "First multimap contents after swap:" << std::endl; for (const auto& elem : a) { std::cout << elem.first << ": " << elem.second << std::endl; } std::cout << "second multimap contents after swap:" << std::endl; for (const auto& elem : b) { std::cout << elem.first << ": " << elem.second << std::endl; } return 0; }
输出
以下是上述代码的输出:
First multimap contents after swap: 3: Hi 4: Hello second multimap contents after swap: 1: Vanakam 2: Namaste
示例
考虑以下场景,我们将交换两个空的 multimap。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; std::multimap<int, std::string> b; a.swap(b); std::cout << "Size of first multimap after swap: " << a.size() << std::endl; std::cout << "SSize of second multimap after swap: " << b.size() << std::endl; return 0; }
输出
上述代码的输出如下:
Size of first multimap after swap: 0 Size of second multimap after swap: 0
multimap.htm
广告