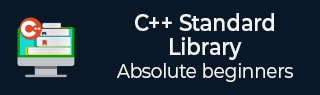
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ multimap::get_allocator() 函数
C++ 的std::multimap::get_allocator()函数用于返回 multimap 用于管理内存的分配器对象。C++ 中的分配器定义了容器元素要使用的内存模型。此函数提供对分配器的访问,从而可以执行自定义内存分配和释放等操作。此函数的时间复杂度为常数,即 O(1)。
语法
以下是 std::multimap::get_allocator() 函数的语法。
allocator_type get_allocator() const noexcept;
参数
它不接受任何参数。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
返回值
此函数返回与 multimap 关联的分配器。
示例
让我们来看下面的例子,我们将演示 get_allocator() 函数的使用。
#include <iostream> #include <map> int main() { std::multimap<int, int> a; auto x = a.get_allocator(); std::pair<const int, int>* y = x.allocate(3); std::cout << "Allocated Memory At: " << y << std::endl; x.deallocate(y, 3); return 0; }
输出
以上代码的输出如下:
Allocated Memory At: 0x55e143b18eb0
示例
考虑以下示例,我们将分配元素数组。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; auto x = a.get_allocator(); auto y = x.allocate(3); for (int i = 0; i < 3; ++i) { x.construct(y + i, std::pair<const int, std::string>(i, "value" + std::to_string(i))); } for (int i = 0; i < 3; ++i) { std::cout << (y + i)->first << " : " << (y + i)->second << std::endl; } for (int i = 0; i < 3; ++i) { x.destroy(y + i); } x.deallocate(y, 3); return 0; }
输出
以下是以上代码的输出:
0 : value0 1 : value1 2 : value2
示例
在下面的示例中,我们将分配和构造多个元素。
#include <iostream> #include <map> int main() { std::multimap<int, int> a; auto x = a.get_allocator(); std::pair<const int, int>* p = x.allocate(2); x.construct(&p[0], std::make_pair(1, 11)); x.construct(&p[1], std::make_pair(2, 22)); for (int i = 0; i < 2; ++i) { std::cout << "Element " << i << ": (" << p[i].first << ", " << p[i].second << ")" << std::endl; } for (int i = 0; i < 2; ++i) { x.destroy(&p[i]); } x.deallocate(p, 3); return 0; }
输出
如果我们运行以上代码,它将生成以下输出:
Element 0: (1, 11) Element 1: (2, 22)
multimap.htm
广告