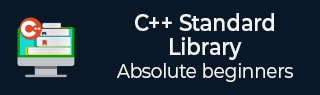
- C标准库
- C标准库
- C++标准库
- C++库 - 首页
- C++库 - <fstream>
- C++库 - <iomanip>
- C++库 - <ios>
- C++库 - <iosfwd>
- C++库 - <iostream>
- C++库 - <istream>
- C++库 - <ostream>
- C++库 - <sstream>
- C++库 - <streambuf>
- C++库 - <atomic>
- C++库 - <complex>
- C++库 - <exception>
- C++库 - <functional>
- C++库 - <limits>
- C++库 - <locale>
- C++库 - <memory>
- C++库 - <new>
- C++库 - <numeric>
- C++库 - <regex>
- C++库 - <stdexcept>
- C++库 - <string>
- C++库 - <thread>
- C++库 - <tuple>
- C++库 - <typeinfo>
- C++库 - <utility>
- C++库 - <valarray>
- C++ STL库
- C++库 - <array>
- C++库 - <bitset>
- C++库 - <deque>
- C++库 - <forward_list>
- C++库 - <list>
- C++库 - <map>
- C++库 - <multimap>
- C++库 - <queue>
- C++库 - <priority_queue>
- C++库 - <set>
- C++库 - <stack>
- C++库 - <unordered_map>
- C++库 - <unordered_set>
- C++库 - <vector>
- C++库 - <algorithm>
- C++库 - <iterator>
- C++高级库
- C++库 - <any>
- C++库 - <barrier>
- C++库 - <bit>
- C++库 - <chrono>
- C++库 - <cinttypes>
- C++库 - <clocale>
- C++库 - <condition_variable>
- C++库 - <coroutine>
- C++库 - <cstdlib>
- C++库 - <cstring>
- C++库 - <cuchar>
- C++库 - <charconv>
- C++库 - <cfenv>
- C++库 - <cmath>
- C++库 - <ccomplex>
- C++库 - <expected>
- C++库 - <format>
- C++库 - <future>
- C++库 - <flat_set>
- C++库 - <flat_map>
- C++库 - <filesystem>
- C++库 - <generator>
- C++库 - <initializer_list>
- C++库 - <latch>
- C++库 - <memory_resource>
- C++库 - <mutex>
- C++库 - <mdspan>
- C++库 - <optional>
- C++库 - <print>
- C++库 - <ratio>
- C++库 - <scoped_allocator>
- C++库 - <semaphore>
- C++库 - <source_location>
- C++库 - <span>
- C++库 - <spanstream>
- C++库 - <stacktrace>
- C++库 - <stop_token>
- C++库 - <syncstream>
- C++库 - <system_error>
- C++库 - <string_view>
- C++库 - <stdatomic>
- C++库 - <variant>
- C++ STL库速查表
- C++ STL - 速查表
C++ New::operator new
在C++中,可以使用new关键字在运行时动态分配RAM堆段中的内存。在声明时,会传递一个指定分配内存大小的参数。可以使用new运算符为预设和唯一的数据类型分配内存。
函数运算符new分配原始内存,在概念上与malloc()相同。
- 它是覆盖默认堆分配逻辑的机制。
- operator new也可以在全局或特定类中重载。
语法
以下是C++ New::operator new的语法:
void* operator new (std::size_t size) throw (std::bad_alloc); (throwing allocation) void* operator new (std::size_t size, const std::nothrow_t& nothrow_value) throw(); (nothrow allocation) void* operator new (std::size_t size, void* ptr) throw();
参数
- size - 包含请求内存块的大小(以字节为单位)。
- nothrow_value - 包含常量nothrow。
- ptr - 指向已分配的适当大小的内存块的指针。
示例1
让我们看一下下面的例子,我们将使用operator new并获取输出。
#include <cstdio> #include <cstdlib> #include <new> void* operator new(std::size_t sz) { std::printf(" new(size_t), size = %zu\n", sz); if (sz == 1) ++sz; if (void *ptr = std::malloc(sz)) return ptr; throw std::bad_alloc{}; } int main() { int* p1 = new int; delete p1; }
输出
让我们编译并运行上面的程序,这将产生以下结果:
new(size_t), size = 4
示例2
让我们看一下另一种情况,我们将使用operator new。
#include <iostream> #include <new> struct MyClass { int data[100]; MyClass() { std::cout << "It constructed [" << this << "]\n"; } }; int main () { std::cout << "1: "; MyClass * p1 = new MyClass; std::cout << "2: "; MyClass * p2 = new (std::nothrow) MyClass; std::cout << "3: "; new (p2) MyClass; MyClass * p3 = (MyClass*) ::operator new (sizeof(MyClass)); delete p1; delete p2; delete p3; return 0; }
输出
运行上述代码后,它将显示如下输出:
1: It constructed [0x5596f5df72c0] 2: It constructed [0x5596f5df7460] 3: It constructed [0x5596f5df7460]
示例3
考虑以下情况,我们将检查operator new的功能。
#include<iostream> #include<stdlib.h> using namespace std; class car { string name; int num; public: car(string a, int n) { cout << "TutorialsPoint" << endl; this->name = a; this->num = n; } void display() { cout << "Name: " << name << endl; cout << "Num: " << num << endl; } void *operator new(size_t size) { cout << "Welcome" << endl; void *p = malloc(size); return p; } }; int main() { car *p = new car("RX100", 2011); p->display(); delete p; }
输出
当代码执行时,它将生成如下输出:
Welcome TutorialsPoint Name: RX100 Num: 2011
广告