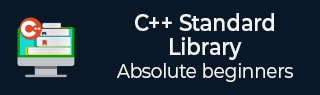
- C标准库
- C标准库
- C++标准库
- C++库 - 首页
- C++库 - <fstream>
- C++库 - <iomanip>
- C++库 - <ios>
- C++库 - <iosfwd>
- C++库 - <iostream>
- C++库 - <istream>
- C++库 - <ostream>
- C++库 - <sstream>
- C++库 - <streambuf>
- C++库 - <atomic>
- C++库 - <complex>
- C++库 - <exception>
- C++库 - <functional>
- C++库 - <limits>
- C++库 - <locale>
- C++库 - <memory>
- C++库 - <new>
- C++库 - <numeric>
- C++库 - <regex>
- C++库 - <stdexcept>
- C++库 - <string>
- C++库 - <thread>
- C++库 - <tuple>
- C++库 - <typeinfo>
- C++库 - <utility>
- C++库 - <valarray>
- C++ STL库
- C++库 - <array>
- C++库 - <bitset>
- C++库 - <deque>
- C++库 - <forward_list>
- C++库 - <list>
- C++库 - <map>
- C++库 - <multimap>
- C++库 - <queue>
- C++库 - <priority_queue>
- C++库 - <set>
- C++库 - <stack>
- C++库 - <unordered_map>
- C++库 - <unordered_set>
- C++库 - <vector>
- C++库 - <algorithm>
- C++库 - <iterator>
- C++高级库
- C++库 - <any>
- C++库 - <barrier>
- C++库 - <bit>
- C++库 - <chrono>
- C++库 - <cinttypes>
- C++库 - <clocale>
- C++库 - <condition_variable>
- C++库 - <coroutine>
- C++库 - <cstdlib>
- C++库 - <cstring>
- C++库 - <cuchar>
- C++库 - <charconv>
- C++库 - <cfenv>
- C++库 - <cmath>
- C++库 - <ccomplex>
- C++库 - <expected>
- C++库 - <format>
- C++库 - <future>
- C++库 - <flat_set>
- C++库 - <flat_map>
- C++库 - <filesystem>
- C++库 - <generator>
- C++库 - <initializer_list>
- C++库 - <latch>
- C++库 - <memory_resource>
- C++库 - <mutex>
- C++库 - <mdspan>
- C++库 - <optional>
- C++库 - <print>
- C++库 - <ratio>
- C++库 - <scoped_allocator>
- C++库 - <semaphore>
- C++库 - <source_location>
- C++库 - <span>
- C++库 - <spanstream>
- C++库 - <stacktrace>
- C++库 - <stop_token>
- C++库 - <syncstream>
- C++库 - <system_error>
- C++库 - <string_view>
- C++库 - <stdatomic>
- C++库 - <variant>
- C++ STL库速查表
- C++ STL - 速查表
C++ New::operator delete[]
C++ New::operator delete[]是一个常规函数,可以像其他任何函数一样显式调用。使用delete[]运算符时,表达式首先调用数组中每个元素的相关析构函数(如果它们是类类型),然后调用数组释放函数。
名为operator delete[]的成员函数执行类对象的数组释放。全局函数operator delete[]用于所有其他情况。当delete[]语句前面带有作用域运算符时,只考虑全局数组释放函数。
语法
以下是C++ New::operator delete[]的语法:
void operator delete[] (void* ptr) throw(); (ordinary delete) void operator delete[] (void* ptr, const std::nothrow_t& nothrow_constant) throw(); (ordinary delete) void operator delete[] (void* ptr, void* voidptr2) throw(); (placement delete)
参数
- size - 包含请求的内存块的大小(以字节为单位)。
- nothrow_value - 包含常量nothrow。
- ptr - 指向已分配的适当大小的内存块的指针。
- voidptr2 - 一个void指针。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
示例1
让我们来看下面的例子,我们将使用operator delete[]并获取输出。
#include <iostream> using namespace std; struct C { C() { cout << "Welcome" << endl; } ~C() { cout << "TP" << endl; } }; int main() { C *p = new C; cout << "pass" << endl; delete[] p; return 0; }
输出
让我们编译并运行上面的程序,这将产生以下结果:
Welcome pass TP TP TP TP TP ........
示例2
让我们来看另一个场景,我们将使用operator delete[]。
#include <iostream> using namespace std; int main() { int *p = new int; cout << "Welcome To World" << endl; delete[] p; return 0; }
输出
运行上面的代码后,它将显示如下输出:
Welcome To World
示例3
考虑以下情况,我们将检查operator delete[]的功能。
#include <cstdio> #include <cstdlib> #include <new> void operator delete[](void* ptr) noexcept { std::puts("TutorialsPoint"); std::free(ptr); } int main() { int* p2 = new int[10]; delete[] p2; }
输出
代码执行后,将生成如下所示的输出:
TutorialsPoint
示例4
让我们考虑另一个例子,我们将使用operator delete[]并获取输出。
#include <iostream> struct MyClass { MyClass() { std::cout <<"MyClass is constructed\n"; } ~MyClass() { std::cout <<"MyClass is destroyed\n"; } }; int main () { MyClass * pt; pt = new MyClass[3]; delete[] pt; return 0; }
输出
代码执行后,将生成如下所示的输出:
MyClass is constructed MyClass is constructed MyClass is constructed MyClass is destroyed MyClass is destroyed MyClass is destroyed
广告