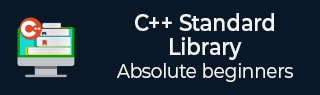
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ Queue::back() 函数
C++ 的std::queue::back()函数用于访问队列的最后一个元素,而无需将其移除。它允许访问最近添加的元素,提供了一种读取或修改该元素的方法。此函数的时间复杂度为O(1)。当需要修改队列中最近添加的元素时,通常会使用此函数。
当此函数在空队列上调用时,会导致未定义行为。
语法
以下是std::queue::back()函数的语法。
reference& back();const_reference& back() const;
参数
此函数不接受任何参数。
返回值
此函数返回对队列中最后一个元素的引用。
示例
让我们来看下面的例子,我们将演示back()函数的基本用法。
#include <iostream> #include <queue> int main() { std::queue<int> a; a.push(11); a.push(22); a.push(33); std::cout << "The last element is: " << a.back() << std::endl; return 0; }
输出
如果我们运行上面的代码,它将生成以下输出:
The last element is: 33
示例
考虑另一种情况,我们将使用back()函数修改最后一个元素。
#include <iostream> #include <queue> int main() { std::queue<int> a; a.push(1); a.push(2); a.back() = 11; std::cout << "After modification the last element is : " << a.back() << std::endl; return 0; }
输出
让我们编译并运行上面的程序,这将产生以下结果:
After modification the last element is : 11
示例
在下面的示例中,我们将back()函数用于循环。
#include <iostream> #include <queue> int main() { std::queue<int> a; for (int x = 1; x <= 5; ++x) { a.push(x * 2); std::cout << "" << a.back() << std::endl; } return 0; }
输出
以上代码的输出如下:
2 4 6 8 10
示例
下面的示例中,我们首先检查队列是否为空,然后向队列添加元素并应用back()函数。
#include <iostream> #include <queue> int main() { std::queue<int> a; if (!a.empty()) { std::cout << "The last element is: " << a.back() << std::endl; } else { std::cout << "The queue is empty" << std::endl; } a.push(1); a.push(2); if (!a.empty()) { std::cout << "After adding elements, the last element is: " << a.back() << std::endl; } return 0; }
输出
如果我们运行上面的代码,它将生成以下输出:
The queue is empty After adding elements, the last element is: 2
queue.htm
广告