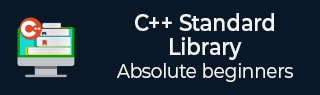
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ Queue::queue() 函数
C++ 的std::queue::queue() 函数构造函数初始化一个队列,这是一个先进先出 (FIFO) 数据结构。默认构造函数 queue() 创建一个空队列,我们可以使用 queue(const Container & cont) 从另一个容器初始化队列。
复制构造函数 queue 和移动构造函数 queue 允许我们从现有队列创建一个新队列,可以通过复制或移动其元素来实现。
语法
以下是 std::queue::queue() 构造函数的语法。
explicit queue (const container_type& ctnr = container_type()); or explicit queue (const container_type& ctnr); or queue( queue&& other); or queue( queue& other );
参数
- ctnr - 它指示容器类型,它是类模板的第二个参数
- other - 它指示另一个相同类型的队列对象。
返回值
构造函数从不返回值。
示例
让我们看看下面的示例,我们将使用默认构造函数。
#include <iostream> #include <queue> int main() { std::queue<int> a; std::cout << "Size of the queue after default construction: " << a.size() << std::endl; return 0; }
输出
以下是上述代码的输出 -
Size of the queue after default construction: 0
示例
考虑另一种情况,我们将使用复制构造函数。
#include <iostream> #include <queue> int main() { std::queue<int> a; a.push(11); a.push(2); std::queue<int> b(a); std::cout << "Size of the queue after copy construction: " << b.size() << std::endl; return 0; }
输出
让我们编译并运行上述程序,这将产生以下结果 -
Size of the queue after copy construction: 2
示例
以下是使用移动构造函数的示例。
#include <iostream> #include <queue> int main() { std::queue<int> a; a.push(111); a.push(22); std::queue<int> b(std::move(a)); std::cout << "Size of the queue after move construction: " << b.size() << std::endl; std::cout << "Size of the queue after move construction: " << a.size() << std::endl; return 0; }
输出
上述代码的输出如下 -
Size of the queue after move construction: 2 Size of the queue after move construction: 0
示例
在下面的示例中,我们将使用不同的容器构造一个队列。
#include <iostream> #include <queue> #include <deque> int main() { std::deque<int> a = {11,22,3,4}; std::queue<int, std::deque<int>> b(a); std::cout << "Queue size: " << b.size() << std::endl; return 0; }
输出
如果我们运行上述代码,它将生成以下输出 -
Queue size: 4
queue.htm
广告