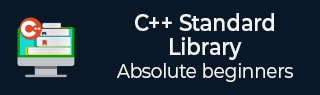
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ Queue::operator<=() 函数
C++ 的std::queue::operator<=()函数用于比较两个队列对象,确定左操作数是否小于或等于右操作数。它根据先进先出 (FIFO) 方式比较元素。如果左队列小于或等于右队列,则返回布尔值 true,否则返回 false。此函数的时间复杂度为线性,即 O(n)。
语法
以下是 std::queue::operator<=() 函数的语法。
bool operator<= (const queue<T,Container>& q1, const queue<T,Container>& q2);
参数
- q1 - 表示第一个队列对象。
- q2 - 表示第二个队列对象。
返回值
如果第一个队列小于或等于第二个队列,则此函数返回 true,否则返回 false。
示例
让我们看看下面的示例,我们将比较队列的大小。
#include <iostream> #include <queue> int main() { std::queue<int> a, b; for (int x = 0; x < 3; ++x) a.push(x); for (int x = 0; x < 5; ++x) b.push(x); if (a.size() <= b.size()) std::cout << "Queue1 is smaller or equal to Queue2."; else std::cout << "Queue1 is larger than Queue2."; return 0; }
输出
以上代码的输出如下:
Queue1 is smaller or equal to Queue2.
示例
在下面的示例中,我们将比较两个队列的队首元素。
#include <iostream> #include <queue> int main() { std::queue<int> a, b; a.push(11); a.push(2); b.push(1); b.push(3); if (a.front() <= b.front()) std::cout << "Front element of queue1 is smaller or equal to front element of queue2. "; else std::cout << "Front element of queue1 is greater than front element of queue2."; return 0; }
输出
以下是以上代码的输出:
Front element of queue1 is greater than front element of queue2.
示例
考虑以下示例,我们将比较队列的队首元素与一个常数值。
#include <iostream> #include <queue> int main() { std::queue<int> a; a.push(1); a.push(22); int x = 5; if (a.front() <= x) { std::cout << "Front element is less than or equal to " << x << std::endl; } else { std::cout << "Front element is greater than " << x << std::endl; } return 0; }
输出
如果我们运行以上代码,它将生成以下输出:
Front element is less than or equal to 5
queue.htm
广告