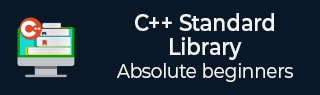
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ Queue::pop() 函数
C++ 的 std::queue::pop() 函数用于移除队列的第一个元素。队列遵循先进先出 (FIFO) 原则,这意味着添加的第一个元素将是第一个被移除的元素。这会使容器大小减一,但不会返回已移除的元素。当尝试在空队列上调用此函数时,会导致未定义的行为。此函数的时间复杂度为常数 O(1)。
此函数与 front() 函数结合使用,以访问第一个元素,然后通过调用 pop() 函数将其移除。
语法
以下是 std::queue::pop() 函数的语法。
void pop();
参数
它不接受任何参数。
返回值
此函数不返回任何值。
示例
让我们来看下面的示例,我们将演示 pop() 函数的基本用法。
#include <iostream> #include <queue> int main() { std::queue<int> x; x.push(11); x.push(222); x.push(3333); std::cout << "Before Pop Front Element:" << x.front() << std::endl; x.pop(); std::cout << "After Pop Front Element:" << x.front() << std::endl; return 0; }
输出
以下是上述代码的输出:
Before Pop Front Element:11 After Pop Front Element:222
示例
考虑下面的示例,我们将使用循环弹出并打印每个元素,直到队列为空。
#include <iostream> #include <queue> int main() { std::queue<int> a; for (int x = 1; x <= 4; ++x) { a.push(x * 2); } while (!a.empty()) { std::cout << "Popping Element: " << a.front() << std::endl; a.pop(); } return 0; }
输出
上述代码的输出如下:
Popping Element: 2 Popping Element: 4 Popping Element: 6 Popping Element: 8
示例
在下面的示例中,我们将使用 pop() 函数后检索队列的大小。
#include <iostream> #include <queue> int main() { std::queue<int> a; a.push(1); a.push(2); a.pop(); std::cout << "Size of the queue after popping:" << a.size() << std::endl; return 0; }
输出
如果我们运行上述代码,它将生成以下输出:
Size of the queue after popping:1
示例
下面的示例将处理队列,直到满足条件。
#include <iostream> #include <queue> int main() { std::queue<int> a; for (int x = 1; x <= 5; ++x) { a.push(x); } while (!a.empty() && a.front() != 3) { std::cout << "Popping Element:" << a.front() << std::endl; a.pop(); } return 0; }
输出
让我们编译并运行上述程序,这将产生以下结果:
Popping Element:1 Popping Element:2
queue.htm
广告