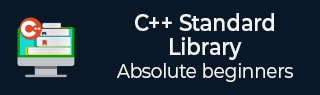
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ Queue::swap() 函数
C++ 的std::queue::swap()函数用于交换两个队列的内容。它作用于包含相同类型元素的队列。它提供了一种高效的方式来交换元素,而无需单独复制它们。
swap() 函数可以通过两种方式调用:作为成员函数或作为非成员函数。当用作成员函数时,swap() 的时间复杂度为常数,即 O(1),否则当用作非成员函数时,时间复杂度为线性,即 O(n)。您可以在下面找到这两种方式的语法。
语法
以下是 std::queue::swap() 函数的语法。
void swap (queue& x) noexcept; or void swap (queue<T,Container>& q1, queue<T,Container>& q2) noexcept;
参数
- x - 表示另一个相同类型的队列对象。
- q1 - 表示第一个队列对象。
- q2 - 表示第二个队列对象。
返回值
此函数不返回任何值。
示例
让我们看下面的例子,我们将交换两个整数队列的内容。
#include <iostream> #include <queue> int main() { std::queue<int> a, b; for(int x = 1; x <= 4; ++x) a.push(x); for(int x = 11; x <= 14; ++x) b.push(x); a.swap(b); std::cout << "Queue 1: "; while (!a.empty()) { std::cout << a.front() << " "; a.pop(); } std::cout << "\nQueue 2: "; while (!b.empty()) { std::cout << b.front() << " "; b.pop(); } return 0; }
输出
以上代码的输出如下:
Queue 1: 11 12 13 14 Queue 2: 1 2 3 4
示例
考虑另一种情况,我们将交换两个字符串队列的内容。
#include <iostream> #include <queue> #include <string> int main() { std::queue<std::string> x, y; x.push("AB"); x.push("BC"); x.push("CD"); y.push("DE"); y.push("EF"); x.swap(y); std::cout << "Queue 1 : "; while (!x.empty()) { std::cout << x.front() << " "; x.pop(); } std::cout << "\nQueue 2 : "; while (!y.empty()) { std::cout << y.front() << " "; y.pop(); } return 0; }
输出
以下是以上代码的输出:
Queue 1 : DE EF Queue 2 : AB BC CD
示例
在下面的示例中,我们将交换空队列和非空队列,并观察输出。
#include <iostream> #include <queue> int main() { std::queue<int> a, b; a.push(11); a.push(222); a.swap(b); std::cout << "Queue 1: "; while (!a.empty()) { std::cout << a.front() << " "; a.pop(); } std::cout << "\nQueue 2: "; while (!b.empty()) { std::cout << b.front() << " "; b.pop(); } return 0; }
输出
如果我们运行以上代码,它将生成以下输出:
Queue 1: Queue 2: 11 222
示例
以下是一个示例,我们将交换空队列,并在交换后检索队列的大小。
#include <iostream> #include <queue> int main() { std::queue<int> a; std::queue<int> b; a.swap(b); std::cout << "Size of queue1 after swap: " << a.size() << std::endl; std::cout << "Size of queue2 after swap: " << b.size() << std::endl; return 0; }
输出
以下是以上代码的输出:
Size of queue1 after swap: 0 Size of queue2 after swap: 0
queue.htm
广告