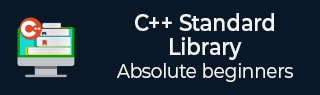
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ Stack::emplace() 函数
C++ 的std::stack::emplace()函数在栈顶构造并插入一个新元素。新元素是就地插入的,即不执行复制或移动操作。此函数接受单个值作为参数,该参数将被转发以在栈中构造新元素。
成员函数emplace()允许在容器(栈)中就地构造对象,而底层容器的成员函数emplace_back()负责实际将对象添加到容器中。因此,emplace()函数有效地调用了带有转发参数的emplace_back()函数。
emplace()函数比push()函数更高效,因为它避免了创建临时对象并将其复制或移动到栈中的开销(使用额外内存)。
语法
以下是std::stack::emplace()函数的语法:
void stack_name.emplace(value);
参数
value - 它是转发用于构造新元素的值。
返回值
此函数不返回值。
示例 1
以下示例演示了std::stack::emplace()函数的用法。首先,我们尝试创建一个栈s。然后,使用for循环使用emplace()函数将五个元素插入到栈中。每个元素都是通过将值“1”与i的当前值相加创建的。最后,我们使用pop()函数弹出栈中的所有元素并将其打印到控制台。
#include <iostream> #include <stack> using namespace std; int main(void) { stack<int> s; for (int i = 0; i < 5; ++i) s.emplace(i + 1); while (!s.empty()) { cout << s.top() << endl; s.pop(); } return 0; }
输出
让我们编译并运行上述程序,这将产生以下结果:
5 4 3 2 1
示例 2
在这里,我们使用emplace()函数在栈顶插入三个简单的字符串并打印它们。
#include <iostream> #include <stack> #include <string> int main() { std::stack<std::string> myStack; // Use emplace() to insert elements into the stack myStack.emplace("first"); myStack.emplace("second"); myStack.emplace("third"); // Print the elements of the stack std::cout << "Elements in stack are: "; while (!myStack.empty()) { std::cout << myStack.top() << std::endl; myStack.pop(); } return 0; }
输出
如果我们运行上面的代码,它将生成以下输出:
Elements in stack are: third second first
示例 3
现在,我们尝试在栈顶插入10的乘法表,然后分别打印它以及栈中存在的元素总数。
#include <iostream> #include <stack> using namespace std; int main(){ stack<int> stck; int total = 0; stck.emplace(10); stck.emplace(20); stck.emplace(30); stck.emplace(40); stck.emplace(50); stck.emplace(60); stck.emplace(70); stck.emplace(80); stck.emplace(90); stck.emplace(100); cout << "Elements in stack are: "; while (!stck.empty()){ cout<<stck.top() << " "; stck.pop(); total++; } cout<<"\nTotal number of elements in stack are: "<<total; return 0; }
输出
上述代码的输出如下:
Elements in stack are: 100 90 80 70 60 50 40 30 20 10 Total number of elements in stack are: 10
示例 4
emplace()函数比push()函数更高效,因为它避免了创建临时对象并将其复制或移动到栈中的开销(使用额外内存)。
在这个例子中,我们定义了一个自定义类MyClass,它带有一个构造函数和复制/移动构造函数,这些函数将消息打印到控制台。然后,我们创建了一个空的MyClass对象栈,并使用emplace()函数和push()函数将三个元素插入到栈中。
使用emplace()函数时,我们将每个元素作为参数提供给函数,我们看到每个元素都是就地创建并插入到栈中的。
使用push()函数时,我们创建MyClass类型的临时对象,然后将其复制或移动到栈中。这需要额外的内存来存储临时对象,并且可能会导致复制或移动对象到栈中的额外开销。
#include <iostream> #include <stack> #include <string> class MyClass { public: MyClass(const std::string& str) : mStr(str) { std::cout << "Constructing MyClass with string: " << mStr << std::endl; } MyClass(const MyClass& other) : mStr(other.mStr) { std::cout << "Copying MyClass with string: " << mStr << std::endl; } MyClass(MyClass&& other) : mStr(std::move(other.mStr)) { std::cout << "Moving MyClass with string: " << mStr << std::endl; } private: std::string mStr; }; int main() { std::stack<MyClass> myStack; std::cout << "Using emplace()" << std::endl; myStack.emplace("first"); myStack.emplace("second"); myStack.emplace("third"); std::cout << "Using push()" << std::endl; MyClass myClass1("first"); MyClass myClass2("second"); MyClass myClass3("third"); myStack.push(myClass1); myStack.push(myClass2); myStack.push(myClass3); return 0; }
输出
执行上述代码时,我们将获得以下输出:
Using emplace() Constructing MyClass with string: first Constructing MyClass with string: second Constructing MyClass with string: third Using push() Constructing MyClass with string: first Constructing MyClass with string: second Constructing MyClass with string: third Copying MyClass with string: first Copying MyClass with string: second Copying MyClass with string: third